In Python, a list is a data type that contains an ordered sequence of objects and is written as a series of comma-separated values between square brackets. Merging lists can be done in many ways in Python.
7 Ways to Merge a List in Python
- Append method
- Extend method
- Concatenation
- Unpacking method
- Itertools.chain
- List comprehension
- For loop
Let’s explore the different methods in detail.
How to Merge a List in Python
1. Append
- The append method will add an item to the end of the list.
- The length of the list will be increased by one.
- It will update the original list itself.
- Return type is
none
.
num1=[1,2,3]
num2=[4,5,6]
num1.append(num2)
print (num1)
#Output:[1, 2, 3, [4, 5, 6]]
2. Extend
- The extend method will extend the list by appending all the items from the iterable.
- The length of the list will increase depending on the length of the iterable.
- It will update the original list itself.
- Return type is
none
.
num1=[1,2,3]
num2=[4,5,6]
num1.extend(num2)
print (num1)
#Output:[1, 2, 3, 4, 5, 6]
3. Concatenation
- Python lists also support concatenation operations.
- We can add two or more lists using the + operator.
- It won’t update the original list.
- Return type is a new
list object
.
Concatenating Two Lists Example
num1=[1,2,3]
num2=[4,5,6]
num3=num1+num2
print (num3)
#Output:[1, 2, 3, 4, 5, 6]
Concatenating Two or More Lists Example
num1=[1,2,3]
num2=[4,5,6]
num3=[7,8]
num4=[9,10]
num5=num1+num2+num3+num4
print (num5)
#Output:[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
4. Unpacking
An asterisk *
denotes iterable unpacking. Its operand must be iterable. The iterable is expanded into a sequence of items, which are included in the new tuple, list or set, at the site of the unpacking.
list1=[*list2,*list3]
First, it will unpack the contents and then create a list from its contents.
num1=[1,2,3]
num2=[4,5,6]
num3=[*num1,*num2]
print (num3)
#Output:[1, 2, 3, 4, 5, 6]
5. Itertools.chain
Itertools.chain is used for creating an iterator from two or more iterables: itertools.chain(*iterables)
. It will accept iterables as an argument. It will return each from the first iterable and then proceed to the next iterable till there are no more iterables left and no more elements to return.
import itertools
num1=itertools.chain([1,2,3],[4,5,6])
#Returns an iterator object
print (num1)#Output:<itertools.chain object at 0x029FE4D8>
#converting iterator object to list object
print(list(num1))#Output:[1, 2, 3, 4, 5, 6]
6. List Comprehension
List comprehension is used to create a new list based on existing iterables. It’s used to write complex functionality in a single line of code. It creates a new list based on the existing values from the iterables.
Syntax for List Comprehension
new_list= [expression for element in iterable if condition]
Joining Two lists Using List Comprehension Example
num1=[1,2,3]
num2=[4,5,6]
num3=[x for n in (num1,num2) for x in n]
print (num3)#Output:[1, 2, 3, 4, 5, 6]
7. For Loop
- The first for loop goes through the lists (for n in
(num1,num2)
. - The second for loop goes through the elements in the list (for x in n)
- Then, it will append each element to the empty list created before
num3
.
num1=[1,2,3]
num2=[4,5,6]
num3=[]
for n in (num1,num2):
for x in n:
num3.append(x)
print (num3)
#Output:[1, 2, 3, 4, 5, 6]
How to Join All Strings in a List
str.join(iterable)
I will join all the elements in the iterable and return a string. We can mention the separator to join the elements in the iterable.
Example 1
In this first example, we’re going to join all strings in the list when the separator is given as space.
num1=["Welcome", "to", "python", "programming", "language"]
num2=" ".join(num1)
print (num2)
#Output:Welcome to python programming language
Example 2
In the second example, we’re going to join all strings in the list when the separator is given as -
.
num1=["1","2","3"]
num2="-".join(num1)
print (num2)
#Output:1-2-3
How to Remove Duplicate Elements While Merging Two Lists
Python sets don’t contain duplicate elements. To remove duplicate elements from lists we can convert the list to set using set()
and then convert back to list using list()
constructor.
num1=[1,2,3,4,5]
num2=[1,3,5,7,9]
num3=list(set(num1+num2))
print (num3)
#Output:[1, 2, 3, 4, 5, 7, 9]
Fastest way to Merge Lists in Python
time.time() → float
Return the time in seconds since the epoch as a floating-point number.
1. Append Method Merge List Time
import time
num1=list(range(1,1000000))
num2=list(range(1000000,2000000))
start=time.time()
num1.append(num2)
print (time.time()-start)
#Output:0.0019817352294921875
2. Extend Method Merge List Time
import time
num1=list(range(1,1000000))
num2=list(range(1000000,2000000))
start1=time.time()
num1.extend(num2)
print (time.time()-start1)
#Output:0.00701141357421875
3. Concatenation Operator Merge List Time
import time
num1=list(range(1,1000000))
num2=list(range(1000000,2000000))
start2=time.time()
num1+num2
print (time.time()-start2)
#Output:0.014112710952758789
Unpacking Method Merge List Time
import time
num1=list(range(1,1000000))
num2=list(range(1000000,2000000))
start3=time.time()
[*num1,*num2]
print (time.time()-start3)
#Output:0.020873069763183594
4. itertools.chain() Merge List Time
import itertools
import time
num1=list(range(1,1000000))
num2=list(range(1000000,2000000))
start4=time.time()
num3=itertools.chain(num1,num2)
list(num3)
print (time.time()-start4)
#Output:0.045874595642089844
5. List Comprehension Merge List Time
import time
num1=list(range(1,1000000))
num2=list(range(1000000,2000000))
start5=time.time()
num3=[x for n in (num1,num2) for x in n]
print (time.time()-start5)
#Output:0.06680965423583984
6. For Loop Merge List Time
import time
num1=list(range(1,1000000))
num2=list(range(1000000,2000000))
start5=time.time()
num3=[]
for n in (num1,num2):
for x in n:
num3.append(x)
print (time.time()-start5)
#Output:0.23975229263305664
Merge List Method Time Comparisons
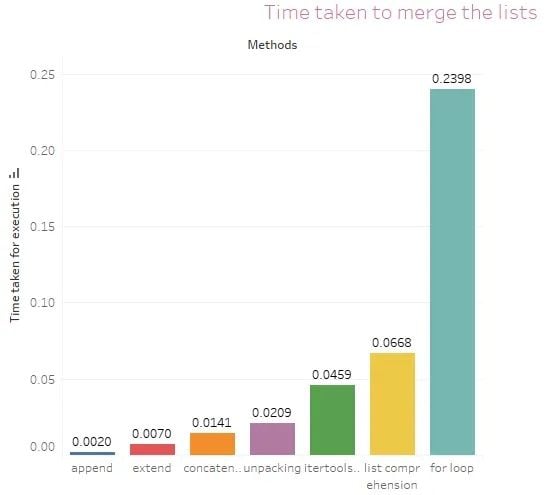
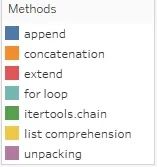
Merging Lists in Python Tips
- The append method will add the list as one element to another list. The length of the list will be increased by one only after appending one list.
- The extend method will extend the list by appending all the items from iterable (another list). The length of the list will increase depending on the length of the iterable.
- Both the append and extend method will modify the original list.
- Concatenation, unpacking and list comprehension returns a new list object. It won’t modify the original list.
- With
itertools.chain()
, the return type will beitertools.chain object
. We can convert to a list using thelist()
constructor.