Python lists and dictionaries are two data structures in Python used to store data. A Python list is an ordered sequence of objects, whereas dictionaries are unordered. The items in the list can be accessed by an index (based on their position) whereas items in the dictionary can be accessed by keys and not by their position.
Let’s see how to convert a Python list to a dictionary.
10 different ways to Convert a Python List to a Dictionary
- Converting a list of tuples to a dictionary.
- Converting two lists of the same length to a dictionary.
- Converting two lists of different length to a dictionary.
- Converting a list of alternative key, value items to a dictionary.
- Converting a list of dictionaries to a single dictionary.
- Converting a list into a dictionary using
enumerate()
. - Converting a list into a dictionary using dictionary comprehension.
- Converting a list to a dictionary using
dict.fromkeys()
. - Converting a nested list to a dictionary using dictionary comprehension.
- Converting a list to a dictionary using
Counter()
.
1. Converting a List of Tuples to a Dictionary
The dict()
constructor builds dictionaries directly from sequences of key-value pairs.
#Converting list of tuples to dictionary by using dict() constructor
color=[('red',1),('blue',2),('green',3)]
d=dict(color)
print (d)#Output:{'red': 1, 'blue': 2, 'green': 3}
2. Converting Two Lists of the Same Length to a Dictionary
We can convert two lists of the same length to the dictionary using zip()
.
zip()
will return an iterator of tuples. We can convert that zip object to a dictionary using the dict()
constructor.
zip()
Make an iterator that aggregates elements from each of the iterables.
“zip(*iterables)
: Returns an iterator of tuples, where the i-th tuple contains the i-th element from each of the argument sequences or iterables. The iterator stops when the shortest input iterable is exhausted. With a single iterable argument, it returns an iterator of 1-tuples. With no arguments, it returns an empty iterator,” according to the Python documentation.
Example
l1=[1,2,3,4]
l2=['a','b','c','d']
d1=zip(l1,l2)
print (d1)#Output:<zip object at 0x01149528>
#Converting zip object to dict using dict() contructor.
print (dict(d1))
#Output:{1: 'a', 2: 'b', 3: 'c', 4: 'd'}
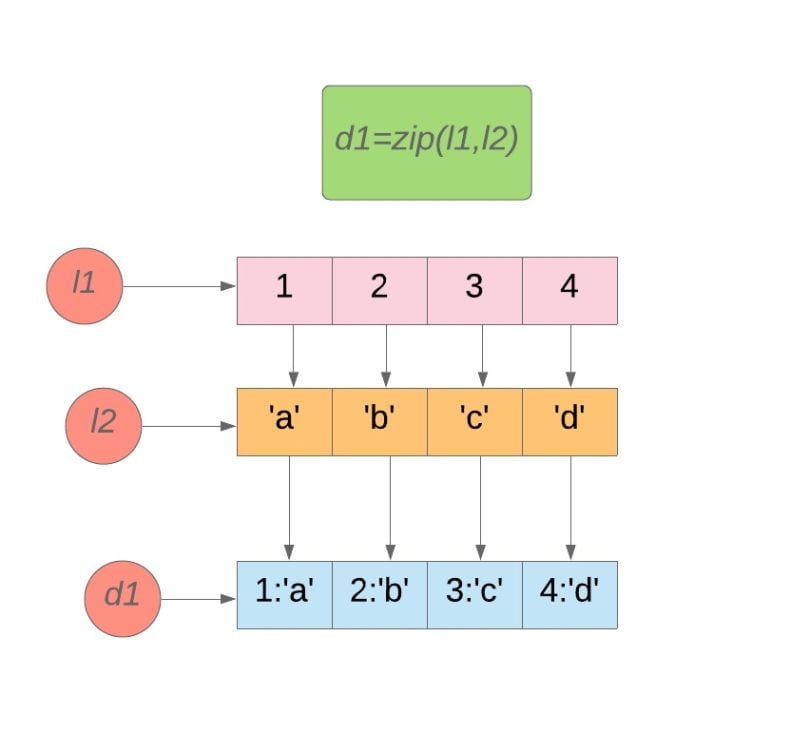
3.Converting Two Lists of Different Length to a Dictionary
We can convert two lists of different length to the dictionary using itertools.zip_longest()
.
According to Python’s documentation, “zip_longest()
: Makes an iterator that aggregates elements from each of the iterables. If iterables are of uneven length, missing value is filled with fillvalue. Iteration continues until the longest iterable is exhausted.”
itertools.zip_longest(*iterables,fillvalue=None)
Using zip()
, iteration continues until the shortest iterable is exhausted.
l1=[1,2,3,4,5,6,7]
l2=['a','b','c','d']
d1=zip(l1,l2)
print (d1)#Output:<zip object at 0x01149528>
#Converting zip object to dict using dict() contructor.
print (dict(d1))
#Output:{1: 'a', 2: 'b', 3: 'c', 4: 'd'}
Using zip_longest()
, iteration continues until the longest iterable is exhausted. By default, fillvalue
is None
.
from itertools import zip_longest
l1=[1,2,3,4,5,6,7]
l2=['a','b','c','d']
d1=zip_longest(l1,l2)
print (d1)#Output:<itertools.zip_longest object at 0x00993C08>
#Converting zip object to dict using dict() contructor.
print (dict(d1))
#Output:{1: 'a', 2: 'b', 3: 'c', 4: 'd', 5: None, 6: None, 7: None}

fillvalue
is mentioned as x.
from itertools import zip_longest
l1=[1,2,3,4,5,6,7]
l2=['a','b','c','d']
d1=zip_longest(l1,l2,fillvalue='x')
print (d1)#Output:<itertools.zip_longest object at 0x00993C08>
#Converting zip object to dict using dict() contructor.
print (dict(d1))
#Output:{1: 'a', 2: 'b', 3: 'c', 4: 'd', 5: 'x', 6: 'x', 7: 'x'}
4. Converting a List of Alternative Keys and Value Items to a Dictionary
We can convert a list of alternative keys and values as items to a dictionary using slicing.
Slicing returns a new list containing a sequence of items from the list. We can specify a range of indexes.
s[i:j:k] — slice of s from i to j with step k
We can create two slice lists. The first list contains keys alone and the next list contains values alone.
l1=[1,'a',2,'b',3,'c',4,'d']
Create two slice objects from this list.
The first slice object will only contain keys:
l1[::2]
start
is not mentioned. By default, it will start from the beginning of the list.
stop
is not mentioned. By default, it will stop at the end of the list.
stop
is mentioned as 2.
l1[::2]
Return a list containing elements from beginning to end using step two (alternative elements).
[1,2,3,4]
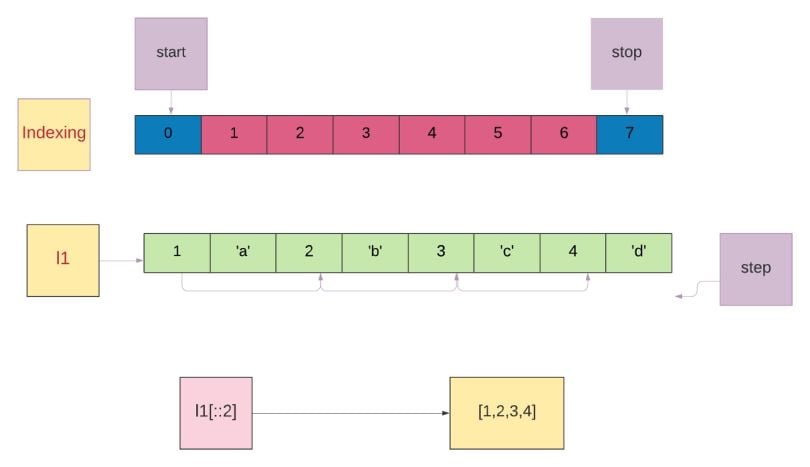
The second slice object will contain values alone.
l1=[1,'a',2,'b',3,'c',4,'d']
l1[1::2]
start
is mentioned as 1. It will start slicing from the first index.
stop
is not mentioned. It will stop at the end of the list.
step
is mentioned as 2.
l1[1::2]
Return a list containing elements from the first index to the end using step 2 (alternative elements).
['a', 'b', 'c', 'd']
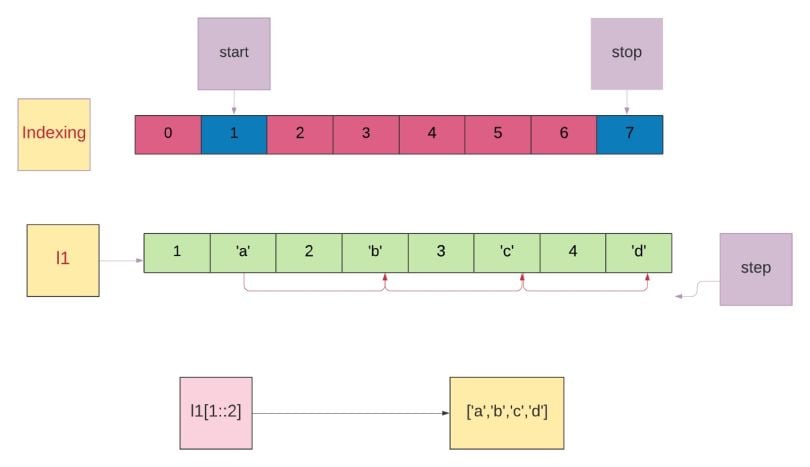
Now we can merge the two lists using the zip()
function.
l1=[1,'a',2,'b',3,'c',4,'d']
#Creating list containing keys alone by slicing
l2=l1[::2]
#Creating list containing values alone by slicing
l3=l1[1::2]
#merging two lists uisng zip()
z=zip(l2,l3)
#Converting zip object to dict using dict() constructor.
print (dict(z))
#Output:{1: 'a', 2: 'b', 3: 'c', 4: 'd'}
5. Converting a List of Dictionaries to a Single Dictionary
A list of dictionaries can be converted into a single dictionary the following ways:
dict.update()
- Dictionary comprehension
Collections.ChainMap
dict.update()
We can convert a list of dictionaries to a single dictionary using dict.update()
.
- Create an empty dictionary.
- Iterate through the list of dictionaries using a
for
loop. - Now update each item (key-value pair) to the empty dictionary using
dict.update()
.
l1=[{1:'a',2:'b'},{3:'c',4:'d'}]
d1={}
for i in l1:
d1.update(i)
print (d1)
#Output:{1: 'a', 2: 'b', 3: 'c', 4: 'd'}
Dictionary Comprehension
A dictionary comprehension consists of brackets{}
containing two expressions separated with a colon followed by a for
clause, then zero or more for
or if
clauses.
l1=[{1:'a',2:'b'},{3:'c',4:'d'}]
d1={k:v for e in l1 for (k,v) in e.items()}
for e in l1:
Return each item in the list {1:’a’,2:’b’}
.
for (k,v) in e.items()
: Return the key, value pair in that item. (1,’a’) (2,’b’)
k:v
Is updated in the dictionary d1.
l1=[{1:'a',2:'b'},{3:'c',4:'d'}]
d1={k:v for e in l1 for (k,v) in e.items()}
print (d1)
#Output:{1: 'a', 2: 'b', 3: 'c', 4: 'd'}
Collections.ChainMap
By using collections.ChainMap()
, we can convert a list of dictionaries to a single dictionary.
“ChainMap
: A ChainMap groups multiple dictionary or other mappings together to create a single, updateable view,” according to Python’s documentation.
The return type will be ChainMap object
. We can convert to a dictionary using the dict()
constructor.
6. Converting a List to a Dictionary Using Enumerate()
By using enumerate()
, we can convert a list into a dictionary with index as key and list item as the value.
enumerate()
will return an enumerate object.
We can convert to dict using the dict()
constructor.
“enumerate(iterable, start=0)
: Returns an enumerate object. iterable must be a sequence, an iterator, or some other object which supports iteration. The__next__()
method of the iterator returned by enumerate()
returns a tuple containing a count (from start which defaults to 0) and the values obtained from iterating over iterable,” according to the Python documentation.
l1=['a','b','c','d']
d1=dict(enumerate(l1))
print (d1)#Output:{0: 'a', 1: 'b', 2: 'c', 3: 'd'}
7. Converting List Into a Dictionary Using Dictionary Comprehension
By using dictionary comprehension, we can convert a list of keys to a dictionary having the same value.
d1={k:"a" for k in l1}
It will iterate through the list and change its item as a key (k
), and value will be a
for all keys.
l1=[1,2,3,4]
d1={k:"a" for k in l1}
print (d1)
#Output:{1: 'a', 2: 'a', 3: 'a', 4: 'a'}
8. Converting a List to a Dictionary Using dict.fromkeys()
dict.from keys()
will accept a list of keys, which is converted to dictionary keys, and a value, which is to be assigned.
The same value will be assigned to all keys.
l1=['red','blue','orange']
d1=dict.fromkeys(l1,"colors")
print (d1)
#Output:{'red': 'colors', 'blue': 'colors', 'orange': 'colors'}
9. Converting a Nested List to a Dictionary Using Dictionary Comprehension
We can convert a nested list to a dictionary by using dictionary comprehension.
l1 = [[1,2],[3,4],[5,[6,7]]]
d1={x[0]:x[1] for x in l1}
It will iterate through the list.
It will take the item at index 0 as key and index 1 as value.
l1 = [[1,2],[3,4],[5,[6,7]]]
d1={x[0]:x[1] for x in l1}
print(d1)#Output:{1: 2, 3: 4, 5: [6, 7]}
10. Converting a List to a Dictionary Using Counter()
“Counter
: Counter is a dict subclass for counting hashable objects. It is a collection where elements are stored as dictionary keys and their counts are stored as dictionary values. Counts are allowed to be any integer value including zero or negative counts,” according to Python’s documentation.
collections.Counter(iterable-or-mapping)
Counter()
will convert list items to keys and their frequencies to values.
from collections import Counter
c1=Counter(['c','b','a','b','c','a','b'])
#key are elements and corresponding values are their frequencies
print (c1)#Output:Counter({'b': 3, 'c': 2, 'a': 2})
print (dict(c1))#Output:{'c': 2, 'b': 3, 'a': 2}