During file processing in Python, you’ll face many situations in which you’ll need to search the index for certain substrings. In this article, I have covered many methods to find the index of the substring in Python.
How to Find the Index of a Substring in Python
- str.find()
- str.rfind()
- str.index()
- str.rindex()
- re.search()
1. str.find()
str.find()
will return the lowest index of the substring mentioned. start
and end
parameters are optional. If start and end parameters are mentioned, it will search the substring within the start
and end
index. It will return -1 if the substring is not found.
str.find(sub,start,end)
Example 1: Using str.find() Method
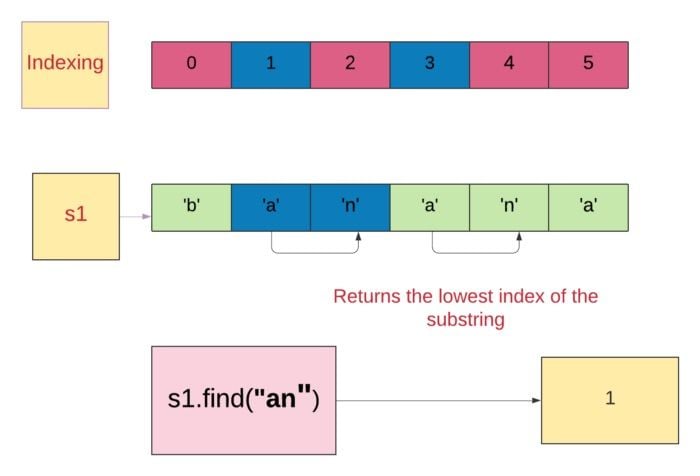
The string is banana
.
The substring is an
.
The substring occurs two times in the string.
str.find(“an”)
returns the lowest index of the substring an
.
s1="banana"
print (s1.find("an"))
#Output:1
Example 2: Using str.find() Method With the Start Parameter
The substring is an
.
The start parameter is 2
. It will start searching the substring an
from index two.
s1="banana"
print (s1.find("an",2))
#Output:3
Example 3: Substring Not found
The substring is ba
.
The start parameter is 1
and the stop parameter is 5
. It will start searching the substring from index 1 to index 5 (excluded).
Since the substring is not found in the string within the given index, it returns -1
.
s1="banana"
print (s1.find("ba",1,5))
#Output:-1
2. str.rfind()
str.rfind()
will return the highest index of the substring mentioned. start
and end
parameters are optional. If start
and end
parameters are mentioned, it will search the substring within the start
and end
index. It will return -1 if the substring isn’t found.
str.rfind(sub,start,end)
Example 1: Using str.rfind() Method
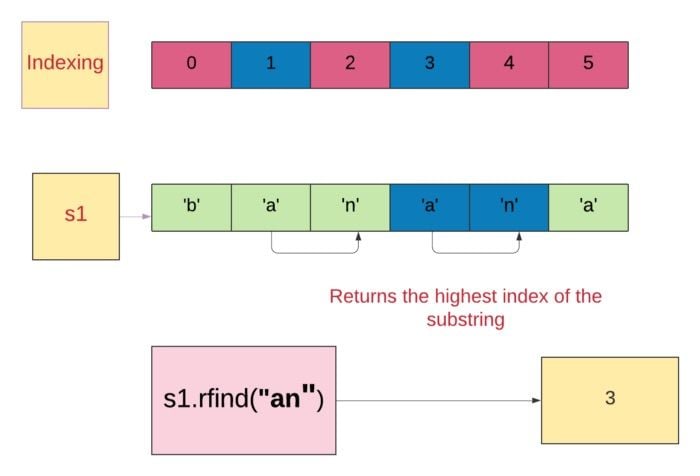
The string is banana
.
The substring is an
.
The substring occurs two times in the string.
str.find(“an”)
returns the highest index of the substring an
.
s1="banana"
print (s1.rfind("an"))
#Output:3
Example 2: Using str.rfind() Method With Start and End Parameters
The substring is an
.
The start and end parameters are 1
and 4
, respectively. It will start searching the substring from index 1 and index 4 (excluded).
s1="banana"
print (s1.rfind("an",1,4))
#Output:1
Example 3: Substring Is Not Found
The substring is no
.
Since the substring is not found in the string, it returns -1
.
s1="banana"
print (s1.rfind("no"))
#Output:-1
3. str.index()
str.index()
will return the lowest index of the substring mentioned. It will raise a ValueError
if the substring is not found.
Example 1: Using str.index() Method
s1="banana"
print (s1.index("an"))
#Output:1
Example 2: Using str.index() Method With Start and End Parameters
s1="banana"
print (s1.index("an",2,6))
#Output:3
Example 3: Substring Is Not Found
s1="banana"
print (s1.index("no"))
#Output:ValueError: substring not found
4. str.rindex()
str.rindex()
will return the highest index of the substring mentioned. It will raise a ValueError
if the substring is not found.
Example 1: Using str.rindex() Method
s1="banana"
print (s1.rindex("an"))
#Output:3
Example 2: Using str.index() Method With Start and End Parameters
s1="banana"
print (s1.rindex("an",0,4))
#Output:1
Example 3: Substring Is Not Found
s1="banana"
print (s1.rindex("no"))
#Output:ValueError: substring not found
5. re.search()
re.search(pattern, string, flags=0)
re.search
(pattern, string): We have to mention thepattern
to be searched in thestring
.- The return type matches the object that contains the starting and ending index of that pattern (substring).
- We can find the
start
andend
indices from the match object usingmatch.start()
andmatch.end()
.
Match.start([group])
Match.end([group])
- We can get the
start
andend
indices in tuple format usingmatch.span(
Match.span([group])
Example 1: Using re.search()
import re
string = 'banana'
pattern = 'an'
match=(re.search(pattern, string))
#Returns match object
print (match)#Output:<re.Match object; span=(1, 3), match='an'>
#getting the starting index using match.start()
print (match.start()) #Output: 1
#Getting the start and end index in tuple format using match.span()
print (match.span()) #Output: (1,3)
Example 2: Substring Is Not Found
import re
string = 'banana'
pattern = 'no'
match=(re.search(pattern, string))
#Returns match object
print (match)#Output: None
What to Know About Each Python String Method
Below is a summary of what you need to remember about each Python string method.
str.find()
,str,index()
: These return the lowest index of the substring.str.rfind()
,str.rindex()
: These return the highest index of the substring.re.search()
: This returns the match object that contains the starting and ending indices of the substring.str.find()
,str.rfind()
: These methods return-1
when a substring is not found.str.index()
,str.rindex()
: These raise aValueError
when a substring is not found.re.search()
: This returnsNone
when a substring is not found.
Frequently Asked Questions
What is the string method Indexof in Python?
The index() method in Python finds the first occurrence of a specific character or element in a string or list. If the character or element is found, its index value will be returned. If not found, the program will return an error.
How do you index a substring in Python?
Finding the index of a substring in Python can be done using any of the following methods:
- str.find()
- str.rfind()
- str.index()
- str.rindex()
- re.search()
Can I slice a string in Python?
A string in Python can be sliced using the slice() method. You can specify which index value in the string to begin slicing, which index to end slicing and how many steps to slice at (if wanting to skip every other character, etc.). The result will return the characters in a string based on the slice specifications.
How do you fetch a substring in Python?
Substrings can be fetched from a larger string in Python using the slice() method. For example, if you want to fetch the substring 'pine' from the string 'pineapple', you could use slice() as follows:
string = "pineapple"
x = slice(0, 4)
print(string[x])