In computer programming, a function is a named section of a code that performs a specific task. This typically involves taking some input, manipulating it and returning an output. In this post, we will go over how to define Python functions, how to specify functions that can take an arbitrary number of positional arguments, and how to specify functions that can take an arbitrary number of keyword arguments.
Let’s get started!
Defining Functions in Python
In computer programming, a function is a named section of a code that performs a specific task. This typically involves taking some input, manipulating it and returning an output
Examples of Functions in Python
To start, let’s define a function that takes two arguments, in this case, two numbers, and returns the sum:
def sum_function(first, second):
return (first + second)
Let’s test our function with the numbers five and 10:
print("Sum: ", sum_function(5, 10))
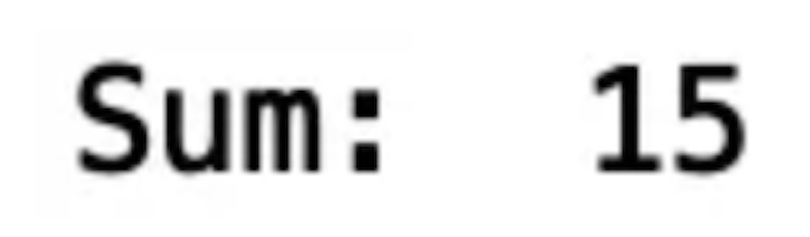
This gives the expected result. We can also use the star expression in Python to pass in an arbitrary number of arguments. For example, we can define a new function:
def sum_arbitrary_arguments(first, *rest):
return (first + sum(rest))
Let’s call this function with the numbers five, 10 and 15:
print("Sum with arbitrary number of arguments: ",
sum_arbitrary_arguments(5, 10,15))

We can call this function with any number of positional arguments. Let’s call the function with five, 10, 15, 20, 25, and 30:
print("Sum with arbitrary number of arguments: ",
sum_arbitrary_arguments(5, 10,15, 20, 25, 30))

This gives the correct value for the sum. We can also specify a function with default keyword arguments. For example, consider a function that, by default, prints the weather in New York:
def print_weather(name, state = "New York", temp = "30 degrees
Celsius"):
print("Hi {}, it is {} in {}".format(name, temp, state))
If we call the function with “Sarah,” we get this:
print_weather("Sarah")

We can also overwrite the default values:
print_weather("Mike", "Boston", "28 degrees Celsius")

We can also write a function that takes an arbitrary number of keyword arguments using the **
expression. Let’s do this for weather reporting:
def print_weather_kwargs(**kwargs):
weather_report = ""
for argument in kwargs.values():
weather_report += argument
return weather_report
We then define a variable “kwargs,” for keyword arguments, as a dictionary and pass it into our function:
kwargs = { 'temp':"30 degrees Celsius ", 'in':'in', 'State':" New
York", 'when': ' today'}
print(print_weather_kwargs(**kwargs))
I’ll stop here, but I encourage you to play around with the code yourself.
Experiment With Functions in Python
To summarize, in this post we discussed how to work with functions in Python. First, we defined a simple function that allows us to find the sum of two numbers, then we showed that we can use the star expression to define a function that can take an arbitrary number of positional arguments. Next, we showed how to define a function with keyword arguments. Finally, we showed how to define a function that can take an arbitrary number of keyword arguments.The code from this post is available on GitHub.