In Python, a function is defined with def
. This is followed by the name of the function and a set of formal parameters. The actual parameters, or arguments, are passed during a function call. We can define a function with a variable number of arguments.
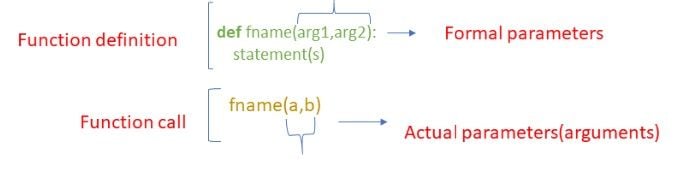
Here’s what you need to know about the five common types of arguments in Python function definition.
5 Arguments in Python to Know
- Default arguments
- Keyword arguments
- Positional arguments
- Arbitrary positional arguments
- Arbitrary keyword arguments
1. Default Arguments in Python
- Default arguments are values that are provided while defining functions.
- The assignment operator
=
is used to assign a default value to the argument. - Default arguments become optional during the function calls.
- If we provide a value to the default arguments during function calls, it overrides the default value.
- The function can have any number of default arguments.
- Default arguments should follow non-default arguments.
In the below example, the default value is given to argument b
and c
.
def add(a,b=5,c=10):
return (a+b+c)
This function can be called in one of three ways:
Giving Only the Mandatory Argument
print(add(3))
#Output:18
Giving One of the Optional Arguments
3 is assigned to a
, 4 is assigned to b
.
print(add(3,4))
#Output:17
Giving All the Arguments
print(add(2,3,4))
#Output:9
Default values are evaluated only once at the point of the function definition in the defining scope. So, it makes a difference when we pass mutable objects like a list or dictionary as default values.
2. Keyword Arguments in Python
Functions can also be called using keyword arguments of the form kwarg=value
.
During a function call, values passed through arguments don’t need to be in the order of parameters in the function definition. This can be achieved by keyword arguments. But all the keyword arguments should match the parameters in the function definition.
def add(a,b=5,c=10):
return (a+b+c)
Calling the function add
by giving keyword arguments
All parameters are given as keyword arguments, so there’s no need to maintain the same order.
print (add(b=10,c=15,a=20))
#Output:45
During a function call, only giving a mandatory argument as a keyword argument. Optional default arguments are skipped.
print (add(a=10))
#Output:25
3. Positional Arguments in Python
During a function call, values passed through arguments should be in the order of parameters in the function definition. This is called positional arguments.
Keyword arguments should follow positional arguments only.
def add(a,b,c):
return (a+b+c)
The above function can be called in two ways:
First, during the function call, all arguments are given as positional arguments. Values passed through arguments are passed to parameters by their position. 10
is assigned to a
, 20
is assigned to b
and 30
is assigned to c
.
print (add(10,20,30))
#Output:60
The second way is by giving a mix of positional and keyword arguments. Keyword arguments should always follow positional arguments.
print (add(10,c=30,b=20))
#Output:60
Default vs. Keyword vs. Positional Arguments
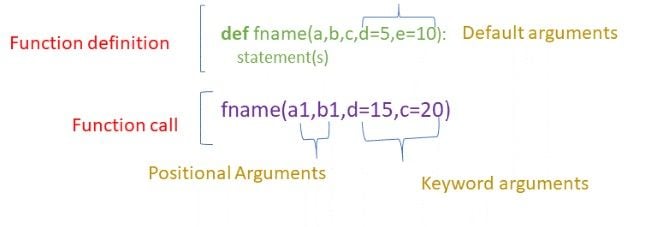
Important Points to Remember for Default, Keyword and Positional Arguments
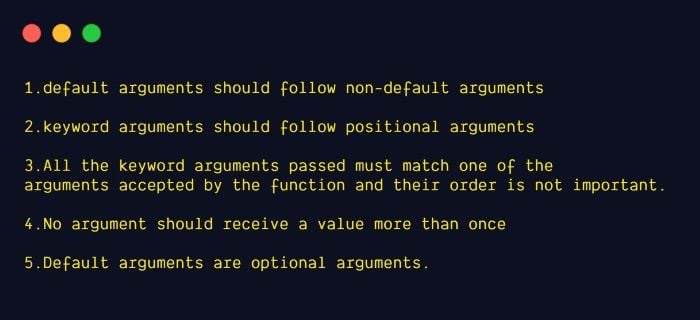
Default Arguments Should Follow Non-Default Arguments
def add(a=5,b,c):
return (a+b+c)
#Output:SyntaxError: non-default argument follows default argument
Keyword Arguments Should Follow Positional Arguments
def add(a,b,c):
return (a+b+c)
print (add(a=10,3,4))
#Output:SyntaxError: positional argument follows keyword argument
All Keyword Arguments Passed Must Match One of the Arguments Accepted by the Function, and Their Order Isn’t Important
def add(a,b,c):
return (a+b+c)
print (add(a=10,b1=5,c=12))
#Output:TypeError: add() got an unexpected keyword argument 'b1'
No Argument Should Receive a Value More Than Once
def add(a,b,c):
return (a+b+c)
print (add(a=10,b=5,b=10,c=12))
#Output:SyntaxError: keyword argument repeated
Default Arguments Are Optional Arguments
Giving only the mandatory arguments:
def add(a,b=5,c=10):
return (a+b+c)
print (add(2))
#Output:17
Giving all arguments (optional and mandatory arguments)
def add(a,b=5,c=10):
return (a+b+c)
print (add(2,3,4))
#Output:9
4. Arbitrary Positional Arguments in Python
Variable-length arguments are also known as arbitrary arguments. If we don’t know the number of arguments needed for the function in advance, we can use arbitrary arguments. Arbitrary arguments come in two types: arbitrary positional arguments and arbitrary keyword arguments.
For arbitrary positional argument, an asterisk (*) is placed before a parameter in function definition which can hold non-keyword variable-length arguments. These arguments will be wrapped up in a tuple. Before the variable number of arguments, zero or more normal arguments may occur.
def add(*b):
result=0
for i in b:
result=result+i
return result
print (add(1,2,3,4,5))
#Output:15
print (add(10,20))
#Output:30
5. Arbitrary Keyword Arguments in Python
For arbitrary keyword argument, a double asterisk (**) is placed before a parameter in a function which can hold keyword variable-length arguments.
def fn(**a):
for i in a.items():
print (i)
fn(numbers=5,colors="blue",fruits="apple")
'''
Output:
('numbers', 5)
('colors', 'blue')
('fruits', 'apple')
'''
Understanding Special Parameters in Python
According to Python Documentation:
“By default, arguments may be passed to a Python function either by position or explicitly by keyword. For readability and performance, it makes sense to restrict the way arguments can be passed so that a developer need only look at the function definition to determine if items are passed by position, by position or keyword, or by keyword.”
As a result, a function definition may look like this:
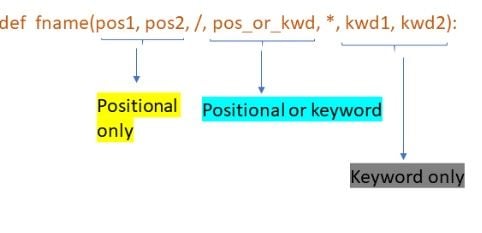
Where /
and *
are optional. If used, these symbols indicate the kind of parameter by how the arguments may be passed to the function, including:
- Positional or keyword arguments.
- Positional only parameters.
- Keyword-only arguments.
Positional or Keyword Arguments
If /
and *
are not present in the function definition, arguments may be passed to a function by position or by keyword.
def add(a,b,c):
return a+b+c
print (add(3,4,5))
#Output:12
print (add(3,c=1,b=2))
#Output:6
Positional Only Parameters
Positional-only parameters are placed before a /
(forward-slash) in the function definition. The /
is used to logically separate the positional-only parameters from the rest of the parameters. Parameters following the /
may be positional-or-keyword or keyword-only.
def add(a,b,/,c,d):
return a+b+c+d
print (add(3,4,5,6))
#Output:12
print (add(3,4,c=1,d=2))
#Output:6
If we specify keyword arguments for positional only arguments, it will raise TypeError.
def add(a,b,/,c,d):
return a+b+c+d
print (add(3,b=4,c=1,d=2))
#Output:TypeError: add() got some positional-only arguments passed as keyword arguments: 'b'
Keyword Only Arguments
To mark parameters as keyword-only, place an *
in the arguments list just before the first keyword-only parameter.
def add(a,b,*,c,d):
return a+b+c+d
print (add(3,4,c=1,d=2))
#Output:10
If we specify positional arguments for keyword-only arguments it will raise TypeError.
def add(a,b,*,c,d):
return a+b+c+d
print (add(3,4,1,d=2))
#Output:TypeError: add() takes 2 positional arguments but 3 positional arguments (and 1 keyword-only argument) were given
All three calling conventions are used in the same function. In the example below, the function add
contains all three arguments:
a
,b
: Positional only arguments.c
: Positional or keyword arguments.d
: Keyword-only arguments.
def add(a,b,/,c,*,d):
return a+b+c+d
print (add(3,4,1,d=2))
#Output:10
Below are some important points to remember for special parameters in Python:
- Use positional-only if you want the name of the parameters to not be available to the user. This is useful when parameter names have no real meaning.
- Use positional-only if you want to enforce the order of the arguments when the function is called.
- Use keyword-only when names have meaning and the function definition is more understandable by being explicit with names.
- Use keyword-only when you want to prevent users from relying on the position of the argument being passed.
Frequently Asked Questions
What are arguments in Python?
In Python, an argument is a value passed to a function during a function call. Arguments are inputs that tell functions what to output, and they allow functions to act in different ways and use different data.
What is the difference between an argument and a parameter?
The terms “argument” and “parameter” are sometimes used interchangeably when referring to Python functions, but they have small differences. Parameters are the variables inside the parentheses of a function, while arguments provide values for parameters and are what’s passed to the function when called.