In this story, I’m going to explain how to display all of the columns and rows of a Pandas DataFrame. I’ll also explain how to show all values in a list inside a DataFrame and choose the precision of the numbers in a DataFrame. And you can do it all with the same tool.
How to Show All Columns and Rows in a Pandas DataFrame
To show all columns in a pandas DataFrame, type:
pd.set_option("display.max_columns", None)
To show all rows in a pandas DataFrame, type:
pd.set_option("display.max_rows", None)
These options will display all columns and rows of a DataFrame when printed.
I’ll be using the top 250 IMDB movies dataset, downloaded from Data World. The database has 250 rows and 37 columns.
pd.set_option(“display.max_rows”, None)
The Problem: Pandas Truncates Information
Sometimes you may read a DataFrame with a lot of rows or columns, but when you display it in Jupyter, the rows and columns are hidden (highlighted in the red boxes):
movies = pd.read_csv("data/IMDB_Top250movies2_OMDB_Detailed.csv")
movies
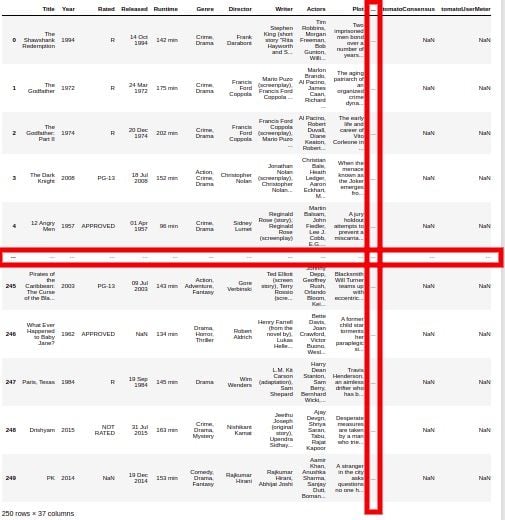
They’re typically hidden to avoid displaying too much information. But sometimes you may want to see all the columns and rows. So, how do we print them all?
To do so, we need to play with the options parameters in Pandas. Let’s see.
How to Show All Columns and Rows in Pandas
I’ll now go over the different commands you can use to manipulate how many columns and rows you can see in Pandas, including:
display.max_columns
max_colwidth
max_rows and min_rows
max_seq_items
Select Your Option
Pandas has an options configuration menu, which allows you to change the display settings of your DataFrame (and more).
All you need to do is select your option with a string name, and get/set/reset the values of it. Those functions accept a regex pattern, so if you pass a substring, it will work, unless more than one option is matched
Display Columns
The display.max_columns
option controls the number of columns to be printed. It receives an int
or None
, the latter used to print all the columns):
pd.set_option('display.max_columns', None)
movies.head()
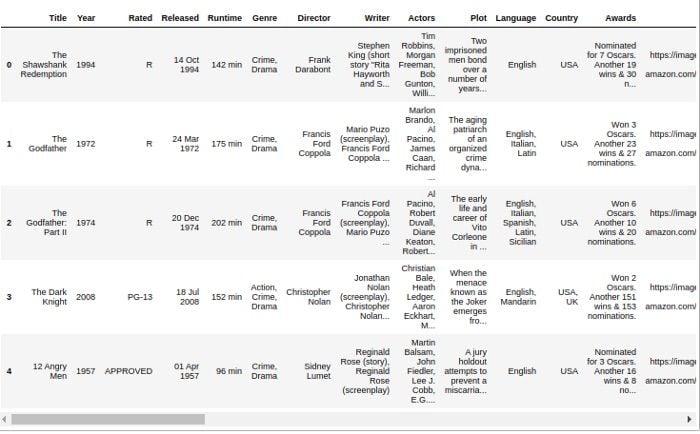
You can also use the string max_columns
instead of display.max_columns
. Remember that it accepts a regex:
pd.set_option('max_columns', None)
To pass a number instead of “None,” enter:
pd.set_option('max_columns', 2)
movies.head()
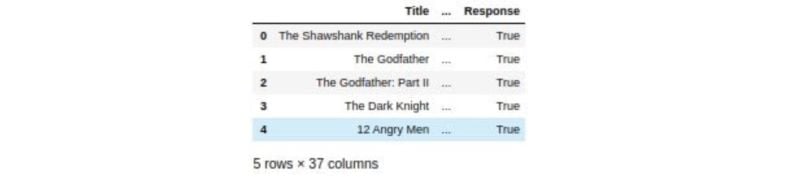
To go back to the default value, you need to reset the option:
pd.reset_option(“max_columns”)
movies.head()
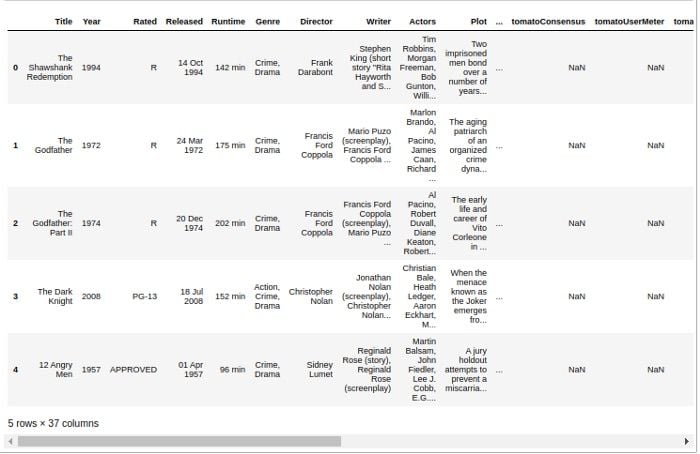
Set the Column Width
You can change the width of the column with the option max_colwidth
. For example, the “plot” column has many characters and the display was originally truncated:
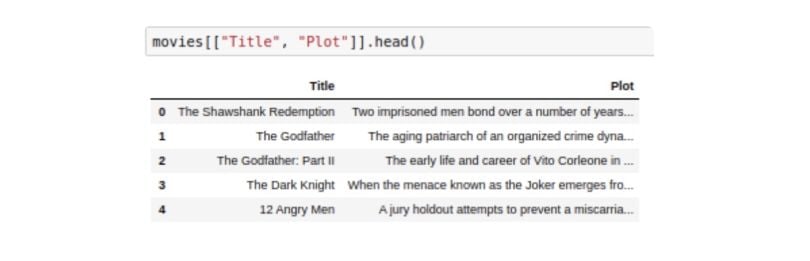
You can increase the width by passing an int
. Or put at the max passing None
:
pd.set_option(“max_colwidth”, None)
movies[[“Title”, “Plot”]].head()
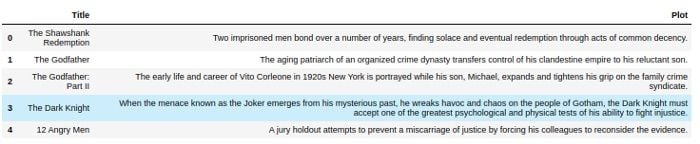
Change the Number of Rows
To change the number of rows you need to change the max_rows
option.
pd.set_option("max_columns", 2) #Showing only two columns
pd.set_option("max_rows", None)
movies
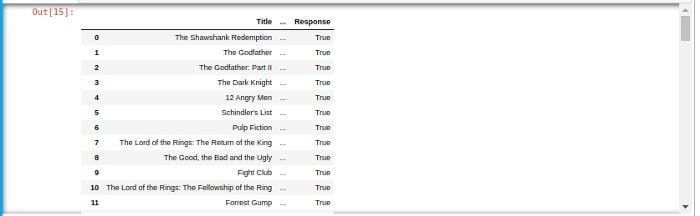
Related to rows, there are two settings: max_rows
and min_rows
. When the number of rows is greater than max_rows
, the DataFrame is truncated and it shows min_rows
rows.
For example, let’s print the movies DataFrame again along with the default values of max_rows
and min_rows
:
print("Default max_rows: {} and min_rows: {}".format(
pd.get_option("max_rows"), pd.get_option("min_rows")))
movies
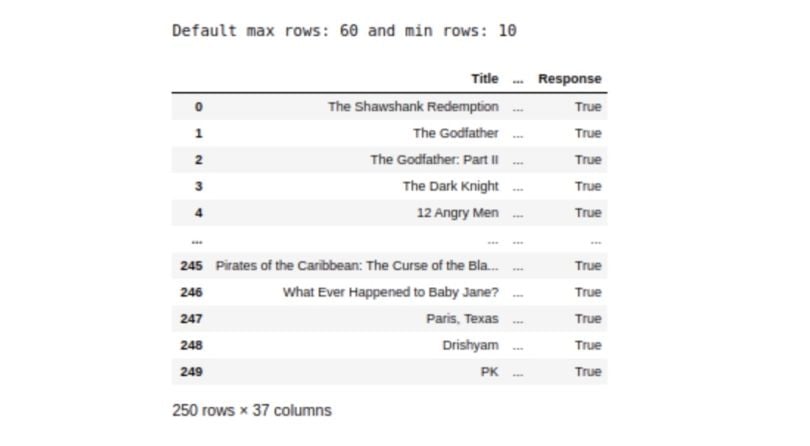
Since the number of rows in the Dataframe is 250, which is more than the max_rows
value 60, it shows the first and last five rows, which is the min_rows
value we set at 10.
If we change min_rows
to two, it will only display the first and the last rows:
pd.set_option(“min_rows”, 2)
movies
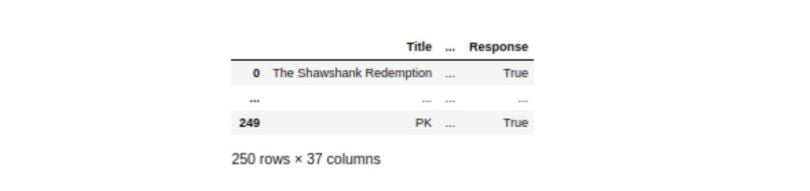
If we use the head
command with a value below the max_rows
value (60), all the rows are shown. For example, using head
with value 20:
movies.head(20)
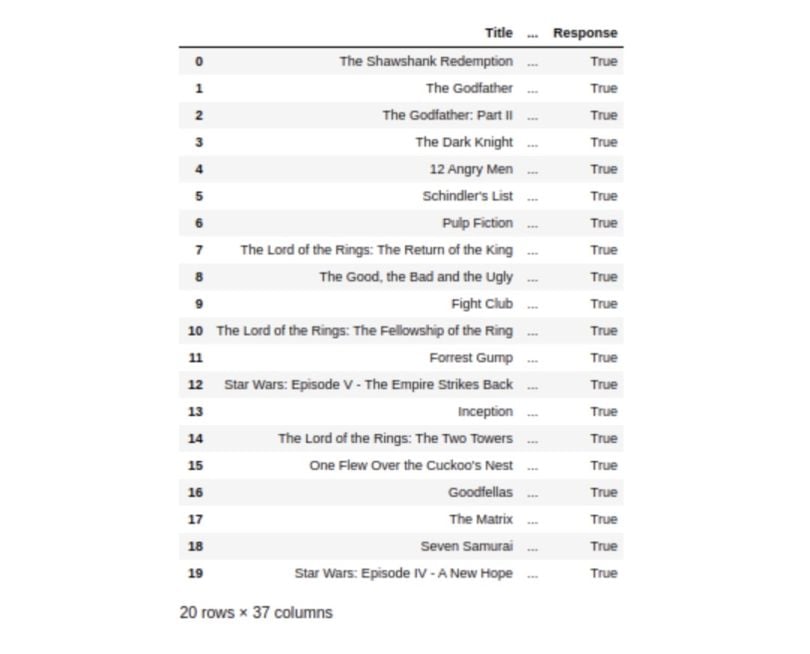
Set the Sequence of Items
The sequence of items (lists) will also be truncated if they have a lot of characters:
#Create "my_list" column and put a list of 100 values in each row
movies[‘my_list’] = [[1]*100] * 250
movies.head()
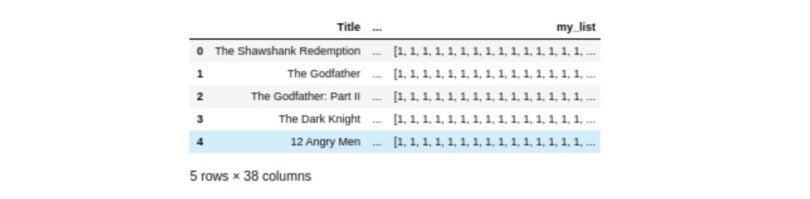
The option to change this behavior is max_seq_items
. But we also have to change the max_colwidth
. If we change the max_colwidth
, the lists will be truncated:
pd.set_option(“max_colwidth”, None)
movies.head()
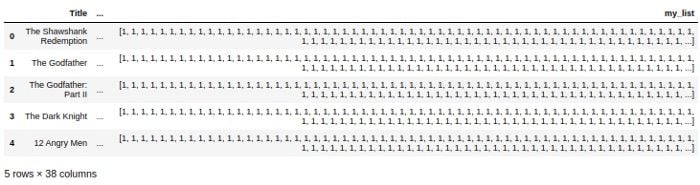
So, you’ll need to change the max_seq_item
.
pd.set_option(“max_seq_item”, None)
movies.head()
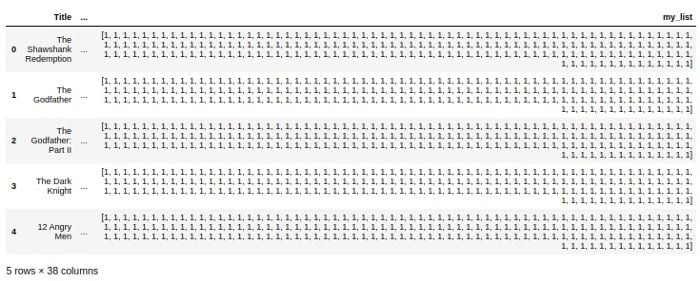
Precision of Numbers in Pandas DataFrame
Another useful option is to set the float precision, or the number of places after the decimal, using the precision option.
#adding more decimal places to imdbRating column
movies[‘imdbRating’] = movies[‘imdbRating’] + 0.11111
movies[[‘imdbRating’]].head()
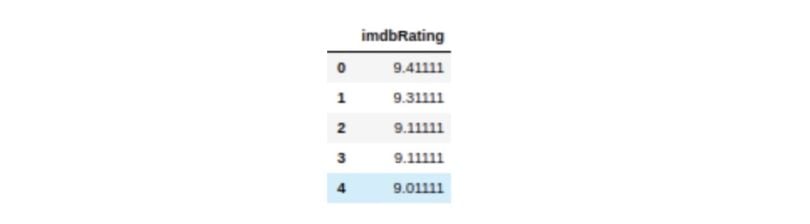
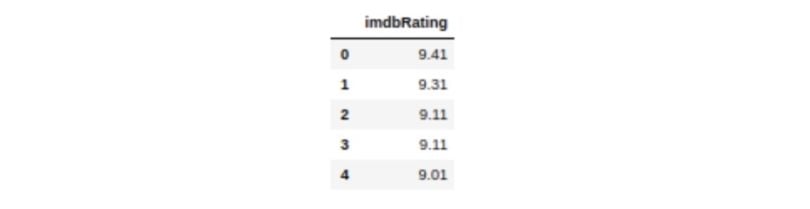
pd.set_option(‘precision’, 2)
movies[[‘imdbRating’]].head()
Frequently Asked Questions
How to show all columns and rows in a Pandas DataFrame
To show all columns and rows in a Pandas DataFrame, do the following:
- Go to the options configuration in Pandas.
- Display all columns with: “display.max_columns.”
- Set max column width with: “max_columns.”
- Change the number of rows with: “max_rows” and “min_rows.”
- Set the sequence of items with: “max_seq_items.”