Everyone has to remove duplicates from an array in JavaScript at some point. It’s even a common coding challenge asked during interviews.
How to Remove Duplicates From a JavaScript Array
- Filter method
- Sets
- forEach method
- Reduce method
- Adding a unique method to the array prototype
- Underscore JS
- Removing duplicate objects using the property name
With that in mind, here are some different ways to filter out duplicates from an array and return only the unique values.
7 Methods to Remove Duplicates From JavaScript Arrays
There are several ways to remove duplicates from a JavaScript array and clean up your code. Below are seven methods for eliminating repeated data from your JavaScript arrays.
1. Filter Method
The filter
method creates a new array of elements that pass the conditional we provide. And any element that fails or returns false
, it won’t be in the filtered array.
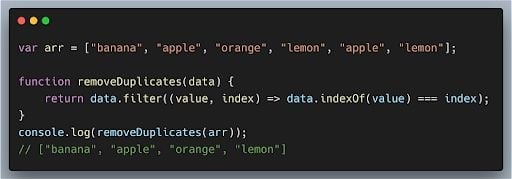
We can also use the filter
method to retrieve the duplicate values from the array by simply adjusting our condition.
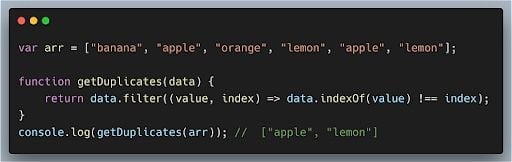
2. Sets
Sets
are a new object type with ES6 (ES2015) that allows you to create collections of unique values.
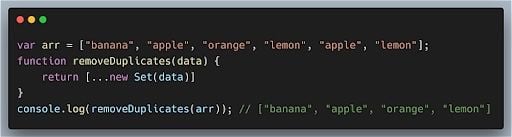
3. forEach Method
By using forEach
, we can iterate over the elements in the array, and we will push into the new array if it doesn’t exist in the array.
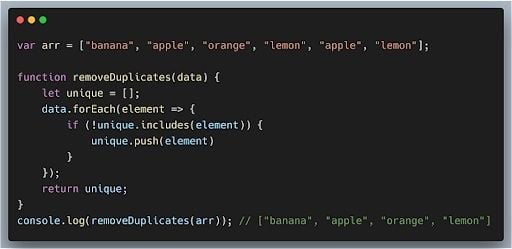
4. Reduce Method
Reduce
is always a bit trickier to understand. The reduce
method is used to reduce the elements of the array and combine them into a final array based on the reducer function that you pass.
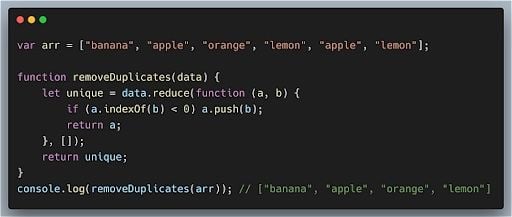
Here is the same reduce
method with a different approach:
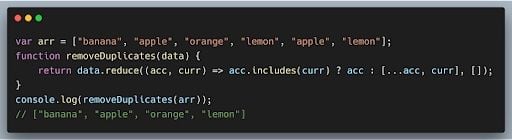
5. Adding a Unique Method to the Array Prototype
In JavaScript, the array prototype constructor allows you to add new properties and methods to the Array
object.
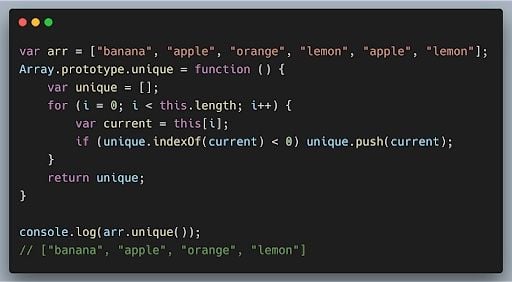
Sets
will work much faster when you compare with the normal approach.
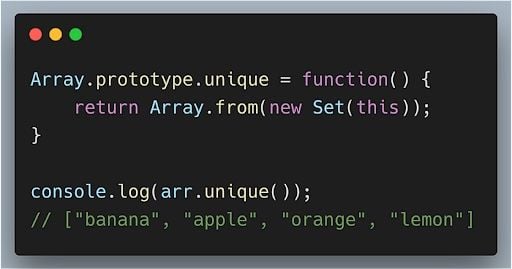
6. Underscore JS
_.uniq
method produces a duplicate-free version of the array. We can also sort this array by passing the second parameter is true
.
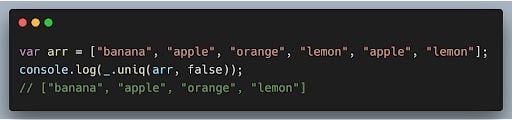
7. Removing Duplicate Objects Using the Property Name
Sometimes you have to remove duplicate objects from an array of objects by the property name. We can achieve this by:
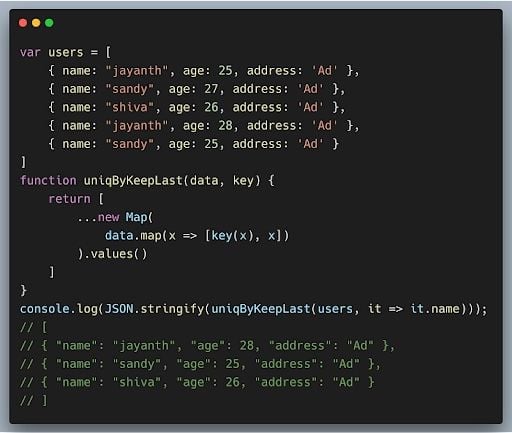