In JavaScript, the filter()
method is an iterative method that calls a callback function once for each element in an array. If the callback function returns true, it includes that element in the return array. The filter()
method is also called a copying method because it returns a shallow copy of an array that contains the same elements as the ones from the original array.
What Does the JavaScript Array Filter() Method Do?
The JavaScript array filter()
method allows you to filter an array to only see elements that meet a specified condition. It uses a callback function to iterate through each element in the array and only returns the ones that meet the specified condition.
The JavaScript filter()
method looks like any other method, except that it accepts parameters that provide more options for manipulating the data within an array.
The filter()
method accepts two named arguments: a callback function and an optional object. The callback function takes three arguments:
currentElement
argument: This is the current element in the array that is being processed by the callback function.- The
Index
of thecurrentElement
that is being processed by the callback function. - The array object.
The index of the array arguments are optional.
JavaScript Array Filter() Syntax
The filter()
method creates a new array with all elements. There are three different ways to write the syntax of filter()
method. The syntax is as follow:
- Arrow function:
filter((element, index)) => {// function body}
- Callback function:
filter(callbackFn, thisArg))
- Inline callback function:
filter(( function(element, index) => {// function body})
callbackFn
executes each element of an array. It returns a truthy value to keep the element in an array. The callbackFn
is called with the following arguments:
element
: The current element being processed in the array.index
: The index of the current element.array
: The arrayfilter()
was called upon.thisArg
(optional): A value to use as this when executingcallbackFn
.
How to Use the JavaScript Array Filter() method
To use the filter()
method in JavaScript, we need to follow four steps:
- Define the array with elements.
- Call the
filter()
method on the array. - Pass the function that will test each element of the array. The function should return true, if the element should be included in the new filtered array.
- Assign the new filtered array to a new variable.
JavaScript Filter() Method Example
Here is an example that uses the filter()
method to filter an array based on a search criteria for car brands that start with the letter “B.”
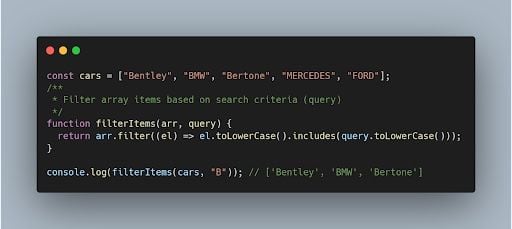
In this example, filter()
method is called on the array cars
to return only car brands that start with the letter “B.” The function filterItems
takes two parameters arr
and query
that returns the new array with matching query value. Inside the filterItems
method, the filter()
method is called on the cars
array, which will filter the array based on the query.
The filter()
method traverses the array and tests each element to determine if it matches with the query. It returns a new array with three brands: Bentley, BMW and Bertone.
The filter()
method does not modify the original array, but instead returns a new array with only the elements that pass the test inside the function.
Using JavaScript Filter() on an Array of Numbers
The following is the code that filters an array of numbers and creates a new array with only the even elements. The steps to use the filter()
on array of numbers are:
- Define the array of numbers.
- Call the filter method on numbers and return the even elements from the array.
- Console the new array to check the output.
Here is an example that demonstrates the program.
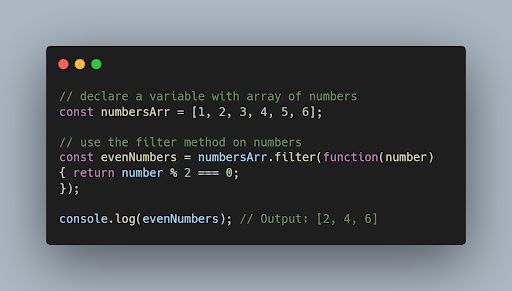
In the above code, we defined an array of numbers numbersArr
. We used the filter()
method on numbersArr
and passed a function that determined if each element is even by using the modulo operator %
to see if the number is divisible by two. If the number is even, the function returns true and the element is included in the new array evenNumbers
. If the number is odd, the function returns false, and the number is excluded from the new array.
Using JavaScript Filter() on an Array of Objects
The following is the code that filters an array of objects and creates a new array with only the truthy elements. The steps to use the filter()
on array of objects are:
- Define the array of objects.
- Call the
filter()
method on array and return the new array that contains only the elements that matches the condition. - Console the new array to check the output.
Here is an example code snippet that demonstrate the program.

In the above code, we defined an array of objects freelancers
. We used the filter()
method on the freelancers
array and passed a function that checks if each freelancer is skilled in JavaScript. If the skill matched the condition, the object was included in the new array filter_freelancers
. If the skill does not match, the function returns false, and the object is excluded from the new array. The result returns a new array with two freelancers, Harry and David, who are skilled in JavaScript.
Using JavaScript Filter() to Find All Prime Numbers
The following is code that filters all the prime numbers from an array and creates a new array with only the prime numbers. The steps to use the filter()
are:
- Define the array of numbers.
- Call the filter() method on array and return the new array that contains only the prime numbers.
- Console the new array to check the output.
Here is an example code snippet that demonstrates the example.
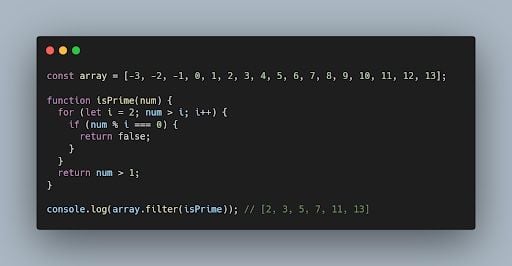
In the above code, we defined an array of numbers array
. We used the filter()
method on array
and passed a function isPrime
that checks if each element is prime. If the number is prime, the function returns true, and the element is included in a new array. If it doesn’t match, the function returns false, and the object is excluded from the new array. The search criteria surfaced a new array with the numbers [2, 3, 5, 7, 11, 13]
.
JavaScript Array Filter() Method Useful Tips
Here are some useful tips to use filter()
method in JavaScript:
- Javascript filter() method always returns a new array, it does not modify the original array.
- Arrow functions provide a concise syntax for defining callback functions. It makes the code easier to read and write.
- Use the second argument. The
filter()
method has an optional second argument that can be used to set the value of this within the callback function. - Chain methods: The
filter()
method can be chained with other array methods likemap()
and reduce() to aggregate data as needed.
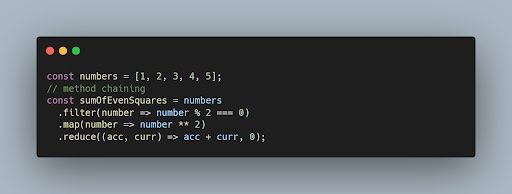
- In the above example, the
filter()
method is used to create a new array with only the even numbers, themap()
method is used to square each even number, and thereduce()
method is used to sum the squared numbers. - Reuse the callback function. It can help to reuse the code and reduce code duplication.
JavaScript Filter() Method Mistakes to Avoid
Here are some common mistakes to avoid when using the filter()
method in Javascript:
- Not returning a boolean value. The
filter()
method expects a function that returns a boolean value. - Mutating the original array. Keep in mind that the
filter()
method does not modify the original array. - Using the wrong syntax. Make sure you’re writing the correct syntax while using
filter()
method. - Failing to handle empty arrays. We need to make sure that we are writing edge cases to handle empty arrays.
- Forgetting to use the
'return'
keyword. If you forgot to use thereturn
keyword in your filter function, the result will be undefined.
Frequently Asked Questions
What does the JavaScript array filter() method do?
The filter() method in JavaScript creates a new array only with elements that pass a test from a given function. The new array made from filter() is a shallow copy of the original array, where it contains only the filtered elements but both arrays still have the same references in memory.
How does a filter work in JavaScript?
In JavaScript, the filter() method uses a callback function or arrow function to iterate through each element in an existing array, and only returns the ones that pass the specified test/conditions. Elements that pass are then placed into a new array. Using filter() doesn’t change the original array, and it doesn’t apply the function to empty elements.