JSON is a human-readable data interchange format developers use to store and transfer data. JSON is an abbreviation for JavaScript Object Notation and pronounced “Jason.”
JSON is language-independent, which means it doesn’t require a specific programming language. This may seem surprising despite its association with JavaScript (the JSON format was inspired by JavaScript object literals). It is, therefore, possible to construct and send JSON messages in one programming language and deconstruct and read those messages in another programming language.
What Data Types Does JSON Support?
JSON’s syntax is text-based and it supports data types such as objects, arrays, strings and numbers. JSON objects are made up of key-value pairs where the keys must be a string, and the values can be any of the supported data types.
An alternative to JSON is Extensible Markup Language (XML). We can use XML like JSON: to transfer or store data for things like configuration files. Whether or not one should be used over the other may come down to the specific services. For example, if the API service is designed to only handle JSON, you are left with no other choice than to use JSON.
What Is JSON Used For?
JSON can be found everywhere on the web today and it is a popular choice of data format to use with RESTful APIs. The APIs can be implemented for both internal and external use, and designed to read and return JSON.
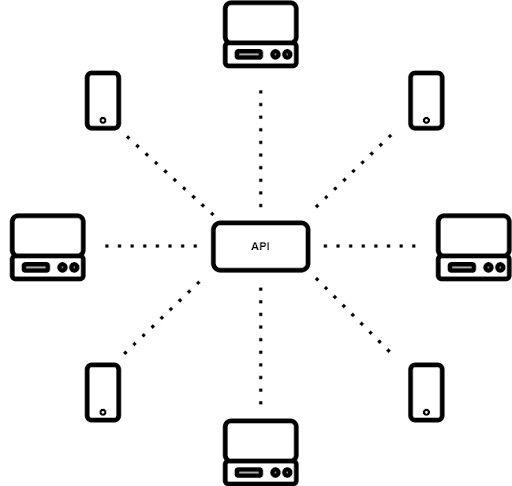
Internal APIs are used by a companies’ own applications while third-party developers or partners can use external APIs. A third-party developer could use a company’s external API to retrieve financial information or product data, like Tweets on Twitter or content on Trello boards, for instance.
Because JSON is language-independent, it does not matter if the back end configuring the company’s the API endpoints is developed in Java or C# and the third-party developer communicates with the API from an application written in JavaScript or Python.
Another important aspect of JSON is that it’s widely supported by many programming languages, which ensures the API endpoints are accessible to as many actors as possible. Some databases, like MySQL, even support JSON as a native data type, which allows us to both store JSON data with automatic validations and later read that data with quick access.
JSON has also other use cases. For instance, you might use JSON to create configuration files to specify metadata to define the name or version number of a particular application.
How Does JSON Work?
We typically pair JSON with a programming language by converting data into a JSON string before we send it to a client that parses the JSON string again.
Let’s say you’re a developer building a to-do list and your users need to access the to-do list from both a mobile app and on a desktop or laptop. This requires your data to be available through online access, which is possible if you store the to-do list’s entries in a database available through a RESTful API.
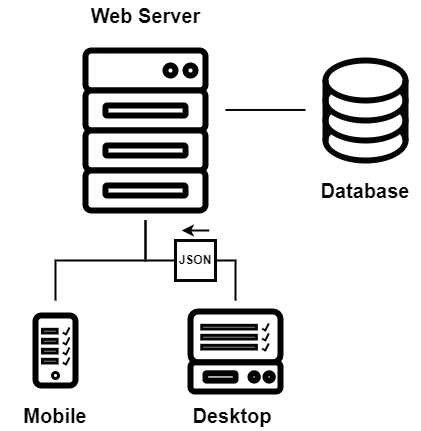
The mobile app and website will retrieve the to-do list’s data as JSON from the server, parse it and display it within each of their to-do list graphical user interfaces (GUI). The clients are also designed to convert the to-do list data into JSON to send the data to the server for storage and updates.
For the sake of this example, let’s say the website can handle retrieving and sending JSON by using JavaScript. JavaScript provides methods such as JSON.stringify()
to convert objects into a JSON string, and JSON.parse()
to parse the JSON string back into objects again.
However, this scenario also involves sending an HTTP (Hypertext Transfer Protocol) request, which you can do in JavaScript by using the Fetch API. The response interface of the Fetch API includes a method called json()
that can parse the JSON string returned from the API into a JavaScript object.
Practicing With JSON
You can find a simple JavaScript example below with a method, called getJSON()
, which is responsible for initiating the HTTP request and parsing the JSON response. The method is designed with the Fetch API and the json()
method. It also shows a sample response (represented as a JavaScript object) that you might receive from the hypothetical to-do list API.
async function getJSON(url) {
const response = await fetch(url);
console.log(await response.json());
}
getJSON(’https://api.example.com/list’);
/* Response:
{
count: 3,
tasks: [
{id: 1, description: “to-do 1”},
{id: 2, description: “to-do 2”},
{id: 3, description: “to-do 3”}
]
}
*/
JSON Syntax and Rules
JSON syntax is similar to JavaScript’s object literals. In fact, key-value pairs in JSON are also called objects.
JSON Key-Value Pairs
JSON also supports similar data types as the ones available in JavaScript: string, number, object, array, true, false and null.
You can construct a valid JSON text using all the supported data types. That said, combining multiple data types on the root level is impossible. For example, a JSON text cannot be combined a string and a number, as you can see.
“This is a string”
345
However, if the string and the number are enclosed by square brackets and separated by a comma, you can format it as a valid JSON array. As with JavaScript, JSON arrays can consist of multiple data types as in the example below.
[”This is a string”, 345]
JSON arrays can specify any of the supported data types, including itself. So, it is possible to create arrays containing other arrays. Like the JSON array, the value of JSON objects can also specify any of the supported data types, but the key must be a string . Here’s an example of a JSON object.
{”key”: 2}
In JSON, you can format numbers as decimals as well as in scientific notation. Your numbers can also be positive or negative (represented with a minus sign). The only real limitation here is that JSON numbers are not allowed to have a leading zero. 092
is not a valid number, for example. Here’s a series of valid JSON numbers.
1
2.3
-43
34E4
22e+2
431e-3
Is JSON Hard to Use?
JSON is not particularly hard to use because there aren’t many prerequisites to using it. It’s human-readable which makes it fairly accessible to even novice developers. JSON also has relatively few data types you need to know before you’re able to use it, so it can be relatively easy to get started using JSON.
The difficulty of using JSON for configuration or data transferring depends on the programming language and your understanding of the required functionality used for the processes. So, whether or not JSON is hard to use may vary depending on the included tools.
However, JSON is widely used and supported in many programming languages. This means there are many JSON tools available. It’s also pretty easy to find information on how to use the format.
JSON: A Brief History
Douglas Crockford first specified JSON in the early 2000s. In 2013, Ecma International standardized JSON and published it in specification ECMA-404. The specification was last updated in 2017, which describes the valid syntax of JSON . Another official specification of JSON, published by Internet Engineering Task Force (IETF), is RFC 8259.