Ruby on Rails (RoR), also referred to as Rails, is a full-stack framework written in Ruby programming language and it is available for multiple operating systems such as Mac OS X, Windows and Linux. The framework is based on the model-view-controller (MVC) pattern and it is shipped with a rich toolkit covering core features regarding both frontend and backend concerns.
Rails is built on a set of predefined patterns, libraries and frameworks that allow beginners and professionals to quickly implement different functionality such as sending mail or reading data from an SQL database. For example, Rails implements an object–relational mapper (ORM) pattern called Active Record, which gives developers the ability to interact with a database using Ruby objects.
What Is Ruby on Rails Used For?
We can use Rails to create web applications and application programming interfaces (APIs), including API-only applications wherein the Rails application only acts as the back end. We can also create applications wherein Rails manages both the back end and front end. Since the release of Basecamp, the first Ruby on Rails application, Rails has been used by many companies and developers to create everything from traditional websites to software-as-a-service (SaaS) applications. This includes companies such as GitHub, Shopify, Zendesk and many others.
Rails’ Active Record is a core part of Rails MVC pattern and is responsible for handling the model behavior. For example, Rails’ Active Record implements the four CRUD operations: create, read, update, delete. Another built-in Rails functionality is the application pre-loader called Spring. Spring ensures the developer can add modifications to the application and automatically see the changes without having to restart background processes.
Rails also gives developers the opportunity to extend the framework with Ruby gems, which is managed by Bundler. Bundler can be configured by a file called Gemfile that specifies download source and the application’s specific Ruby gems.
What Are the Advantages of Ruby on Rails?
One of the major advantages of Rails is its cost efficiency. Rails comes with a more or less complete toolbox providing the tools you need to build web applications and APIs quickly and without much effort. The framework incorporates concepts and patterns saving you valuable time that can be better spent working on the actual solution, instead.
Other advantages are within security. Rails handles many different security aspects, thereby empowering beginners and professionals to build secure applications. Rails is free to use so you don’t have to pay for an expensive license and it has an active community to ensure its continued development. Rails also allows developers to extend the framework, which allows them the opportunity to extend Rails for their specific needs.
See an outline reiterating Rails’ many advantages below.
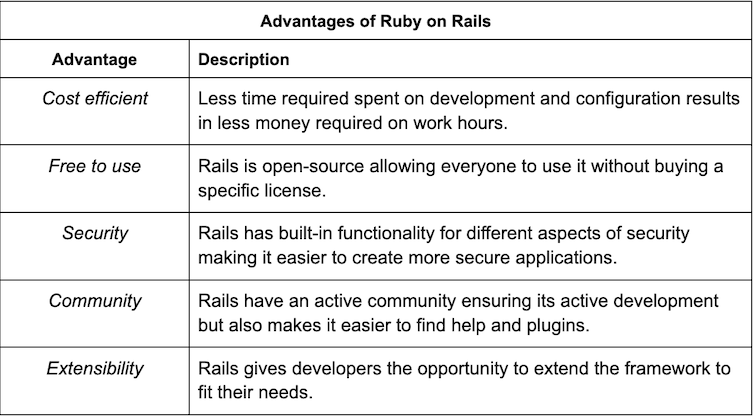
Ruby on Rails Alternatives
Similar web framework alternatives to Rails are Laravel, Sails.js and Django, which share many similarities to Rails. For example, they are all open-source, extendable, database ready frameworks that include an MVC and ORM pattern. Though, I should note that Django’s MVC pattern has been referred to as a model-template-view (MVT) pattern because Django handles the controller layer differently. Despite their similarities, these frameworks are all written in programming languages other than Ruby. Laravel is written in PHP, Sails.js in JavaScript and Django in Python.
Other slightly different web framework alternatives to Rails include the micro frameworks Flask and Sinatra. Flask and Sinatra don’t include a database layer, ORM or MVC, but they are supported through extensions. Flask is written in Python and Sinatra in Ruby.
Here’s a table reiterating the alternatives to Rails:

Is Ruby on Rails Dead?
Many similar frameworks have been launched since Rails’ release in the early 2000s. The growth of viable alternatives might make you wonder if Rails is still relevant.
At the time of writing, Rails is still the backbone for thousands of websites and it has an active community that consistently updates the framework with new features. This year alone, nine version updates have been added to rubygems.org.
Rails has a large share of the market compared to its competitors Laravel, Django and Sails.js. Despite Rails’ large share of the market, the number of websites using Rails decreased between 2020-2021, which makes the difference in adoption between the mentioned frameworks a bit smaller (BuiltWith, 2022a).
Because the continuous updates to the framework contributes to keeping it secure and up-to-date with best online security practices, thousands of websites already use Rails. As the need for SaaS applications continues to grow, one could argue that the framework won’t die anytime soon.
Ruby on Rails Tutorial
The following example describes how to create a simple book review application with Ruby on Rails.
Create a New Application
You can create a new Rails application by typing rails new app path
into a terminal application.
$ rails new BookReview
The rails
command gives access to a list of Rails generators, which you can use for modifications, such as adding code or database migrations. It’s also possible to create custom generators to add custom behavior. To find more information about the available Rails commands, type rails –help
into the terminal.
Generate Review Model, Controller and View With Scaffold
There are several approaches you can take to create models, controllers and views within a Rails application. For example, you can generate a single controller using the command <rails generate controller>
. You can also generate a single model using the command rails generate model <entity name>
.
However, it’s also possible to generate files for both the model, controller and view by using the command rails generate scaffold<entity name>
. Enter the following into the terminal to create the necessary files to add book review functionality to the application.
$ rails generate scaffold Review title:string content:text recommend:boolean
The scaffold command not only creates the necessary files, but also adds methods to the controller, views, RESTful routes and a database migration file you can use to add the model entity as a table in the SQL database.
The files created in the process output to the terminal after executing the command (see below).
invoke active_record
create db/migrate/20220906224020_create_reviews.rb
create app/models/review.rb
invoke test_unit
create test/models/review_test.rb
create test/fixtures/reviews.yml
invoke resource_route
route resources :reviews
invoke scaffold_controller
create app/controllers/reviews_controller.rb
invoke erb
create app/views/reviews
create app/views/reviews/index.html.erb
create app/views/reviews/edit.html.erb
create app/views/reviews/show.html.erb
(...)
Migrate Database
In order to add the table to the database, it’s necessary to migrate the migration file, which was in turn added by the scaffold command. You can do this by entering the command rake db:migrate
into the terminal. Another related and useful command to know is rake db:rollback
, which rolls back the latest migration.
$ rake db:migrate
You can find the migration file under ./db/migrate/
as a Ruby file. The file contains the operations you should execute to the database. For example, as you can see below, the migration file should create the review table with a title
, content
and recommend
property. The last property called timestamps
adds time properties such as created_at
, which saves the time a record is added to the table, and updated_at
, which saves the last time a record was modified.
class CreateReviews < ActiveRecord::Migration[7.0]
def change
create_table :reviews do |t|
t.string :title
t.text :content
t.boolean :recommend
t.timestamps
end
end
end
Adding Routes
We use Rails Router to handle paths and URLs within a Rails application. You can find the configuration file at ./config/routes.rb
. The scaffold command automatically adds RESTful routes mapped to the reviews controller by adding the resources
method to the router. The scaffold command does not specify a root path for the application. You’ll need to configure this manually in the routes.rb
file by using the root
method. The root
method takes a string as an argument specifying controller and controller method. For example, you can specify the reviews controller and its index method by root “reviews#index”
(see the snippet below).
Rails.application.routes.draw do
resources :reviews
root "reviews#index"
# Define your application routes per the DSL in
https://guides.rubyonrails.org/routing.html
# Defines the root path route ("/")
# root "articles#index"
end
Start Server
You’re now ready to test the application locally in a browser. Start the development server by typing rails server
into the terminal. You can access the application by entering localhost:3000
in a browser.
$ rails server
The application already contains everything you need to show, create, edit, update and delete book reviews. You can find the HTML files for the review controller at ./app/views/reviews/
. Here’s an example of the default new review and show review pages.
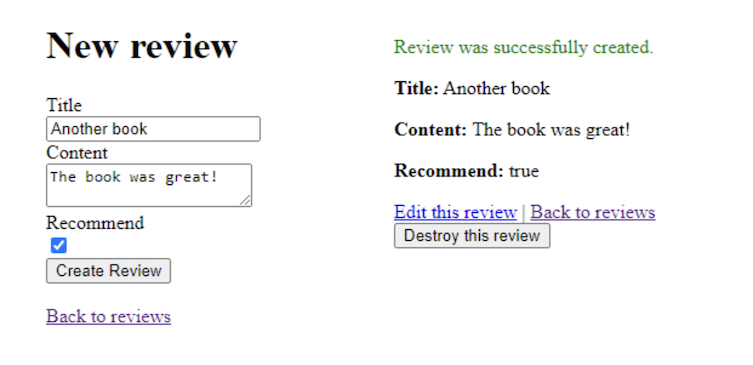
Update the Presentation of a Book Review
You can find the HTML and embedded Ruby (ERB) representing the application’s book reviews as a partial template. Embedded Ruby empowers you to write Ruby directly in a HTML file; a partial template is a functionality used to create reusable HTML files you can render within other HTML files.
You can find the file specifying the HTML and ERB for the book review at ./app/views/reviews/_review.html.erb
. The file currently shows the recommend
property by its value, true
or false
as text. Another approach could be to display a message saying Recommended!
or Not recommended!
based on the recommend
property’s boolean value. You can easily implement this approach with an if-else statement as shown below.
<% if review.recommend %>
I Recommend!
<% else %>
Not recommended!
<% end %>
Another change you can add to the book review is time information. The table created by the migration earlier in the example added two timestamps: created_at
and updated_at
. You can fetch the value of these properties and display them using the same type of notation for other properties (title, content and more). See the snippet below.
<%= review.created_at %>
<%= review.updated_at %>
You can format timestamps by a Ruby method called strftime
. The method takes a string as an argument specifying the way in which the time should be formatted. For example, passing “%d.%m.%Y”
as an argument will output a string with day of the month (%d
), month of the year (%m
) and year with century (%Y
). See the snippet below.
<%= review.created_at.strftime("%d.%m.%y") %>
<%= review.updated_at.strftime("%d.%m.%y") %>
Add the code from the snippet below to the _review.html.erb
in order to implement the changes to the book review’s recommend
property and to add timestamps.
<div id="<%= dom_id review %>">
<h2>
<%= review.title %>
</h2>
<p>
<%= review.content %>
</p>
<p>
<% if review.recommend %>
I Recommend!
<% else %>
Not recommended!
<% end %>
</p>
<small>
Published: <%= review.created_at.strftime("%d.%m.%y") %> |
Last update: <%= review.updated_at.strftime("%d.%m.%y") %>
</small>
</div>
After adding the changes to the reviews partial, the show page should look like the image below.
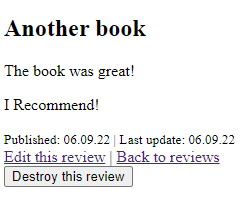
_review.html.erb
file. | Image: Nicolai Berg Andersen
Adding Input Validation
Users are currently able to add new book reviews with empty titles and content. A way to avoid empty input is by adding validations to the Review
model. Rails implements several methods you can use for different types of validations, such as uniqueness, presence and more.
You can use the validates
and validate_presence_of
methods to validate whether or not a property value is present. The difference here is that validates
allows several validation conditions to be added in a single line.
The snippet below shows how to check the presence of the properties title and content.
class Review < ApplicationRecord
validates :title, presence: true
validates :content, presence: true
end
If a user tries to save a book review with an empty title or content after adding the validation, the request will fail. You can find the validation errors by calling the errors
property on the object that failed to save or be modified. The errors
property contains an OrderedHash
where the key specifies the property that failed to validate and the value specifies a string array of error messages.

The form partial at ./app/views/reviews/_form.html.erb
uses the errors
property to display failed validations when creating or editing a book review, as you can see in output above.
Ruby on Rails: A Brief History
Rails was created in 2003 by David Heinemeier Hansson while he was working on Basecamp and working at a web software company named 37Signal. Basecamp, a project management tool, was the first Ruby on Rails application. Ruby on Rails is an open source framework that’s maintained by a community of volunteers known as Rails Core, The Committers and The Issues team.
One of the core philosophical concepts behind Rails is the focus on developer happiness, as described by David Heinemeier Hansson. He calls this the Principle of the Bigger Smile in Rails Doctrine. Other concepts in Rails include Don't Repeat Yourself (DRY) and Convention Over Configuration (CoC).