As a developer, presenting dates in a readable and user-friendly format is crucial for enhancing the user experience. In this comprehensive guide, we’ll dive into various techniques to format dates in JavaScript, exploring different libraries and native methods, and providing code examples for each approach.
3 Ways to Format Dates in JavaScript
- Use JavaScript’s native
date
methods. - Use
Intl.DateTimeFormat
for international dates. - Use JavaScript date libraries for more flexibility.
3 Methods to Format Dates in JavaScript
1. Using JavaScript’s Native Date Methods
JavaScript’s Date
object offers several methods to retrieve individual date components, which you can then assemble into your desired format:
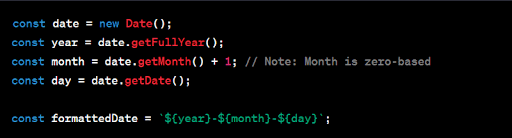
2. Using Intl.DateTimeFormat
Here’s how to present international dates usingIntl.DateTimeFormat
:
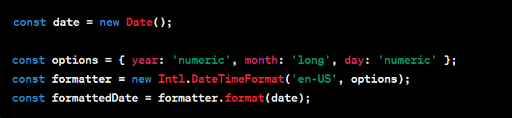
3. Using JavaScript Date Libraries
Using libraries like moment.js
, Deprecated
, date-fns
, Luxon
or Day.js
provides a more advanced and flexible date formatting options:
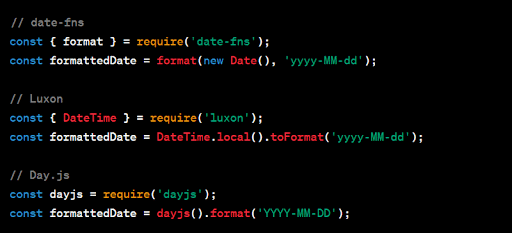
Best Practices to Showing Dates in JavaScript
1. Consider User Locale
When using native methods or Intl.DateTimeFormat
, consider your user’s preferred locale for proper date formatting.
2. Library Selection
While libraries provide powerful formatting capabilities, consider the trade-offs in terms of performance and bundle size.
3. Consistency
Maintain consistent date formats across your application for a better user experience.
Formatting dates in JavaScript is a skill that enhances the usability of your applications. By leveraging JavaScript’s native methods or utilizing specialized libraries, you can easily customize date presentation to match your application’s needs.
Frequently Asked Questions
How do you format dates in JavaScript?
The most common way to format dates in JavaScript is to use the date object:
date.getFullYear()
: Formats the full yeardate.getMonth()
: Returns the month.date.getDate()
: Returns the day.
What JavaScript date libraries are available to format dates?
There are a few common JavaScript date libraries you can use for more flexible date options. These include: moment.js
, Deprecated
, date-fns
, Luxon
and Day.js
.