React is one of the most popular front-end JavaScript libraries, appearing everywhere from e-commerce sites to web-based tools. At its core, React is a declarative, component-based, open-source library that was developed and maintained by Meta. First released in 2013, React has become a fan favorite among developers for its flexibility and unique features like reusable components and virtual DOM.
What Is React Used For?
React is known for its high performance, enabling developers to update page content dynamically without requiring a full page reload. It also uses a component-based, which promotes reusability, reducing repetitive code and helping create consistent and maintainable user interfaces.
How Does React Work
React simplifies user interface development by combining three key ideas: declarative programming, component-based architecture and an efficient Virtual DOM system.
Declarative Programming
There are two paradigms in programming we often hear about: declarative and imperative. Imperative programming is writing the actual steps for how something should behave, whereas declarative just expresses how something should behave without the programmer needing to specify the individual steps.
Consider these two pieces of code. In the first, we can see that we have to specify each step to create the HTML we want.
// Imperative
export const addHeading = () => {
// create the heading
const heading = document.createElement('h2')
// fill it with the text we want
heading.textContent = 'Hello, world';
// add the style we want
heading.style.color = "pink"
// add it to the page
document.body.appendChild(heading)
}
In the second, we have a React component, which accomplishes much the same thing without having to explicitly write out each step. We can just tell React what our HTML should look like and it’ll go ahead and create that.
// Declarative
export const Heading = () => {
return <h1 style={{ color: "pink" }}>Hello, world!</h1>;
};
Component-Based Architecture
React’s declarative style is built around components, a combination of HTML and (sometimes) JavaScript code that can be reused and combined like building blocks. A component can be something small like a button or a larger item like a page section. All web pages have some repeated elements. React allows you to build that component once and reuse it in different scenarios.
For example, if we’re recreating Jeni’s ice cream flavors page using React, we can consider each of the highlighted color areas a separate component we could build once and reuse (there are likely many more on the page that aren’t highlighted).
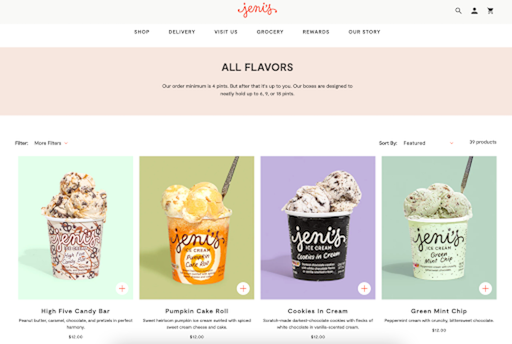
Components use data passed into them in order to render additional content in different scenarios, and are often composed of multiple components themselves. We can see an example of that with the ice cream card component (highlighted in pink), which has a plus sign button component inside it (highlighted in blue
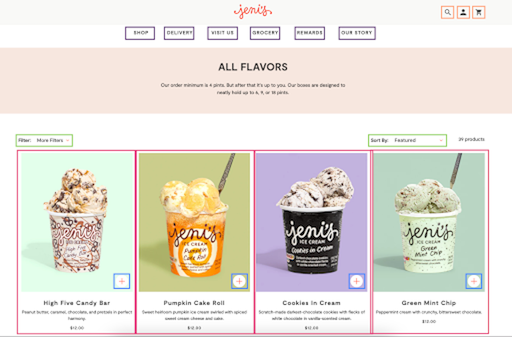
Virtual DOM
One of React’s performance advantages lies in its use of the Virtual DOM. Direct manipulation of Document Object Model (DOM) can be computationally expensive, particularly when many elements require updates.
This is where the Virtual DOM comes into play. As we saw in the explanation of declarative programming above, React takes away the need to write code that directly manipulates the DOM. Instead, React creates a copy of the actual DOM. Any change to the HTML that should happen due to some change in data first creates another copy of the Virtual DOM. React then compares the new copy with the old and batches only the needed updates to the real DOM.
Due to the powerful diffing algorithms that React employs, it’s possible to see frequent DOM manipulations and re-rendering without performance suffering.
How to Create a Web App With React
React created a syntax for writing HTML with Javascript called JSX. We can use it to compose components into a hierarchy that represents the display we want.
Let’s look at the Jeni’s page again.
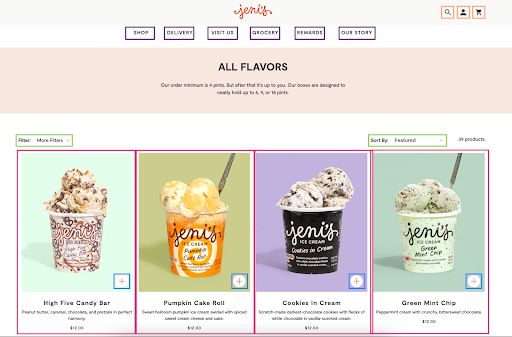
If we were to represent this (loosely) with a React component tree, it might look something like this:
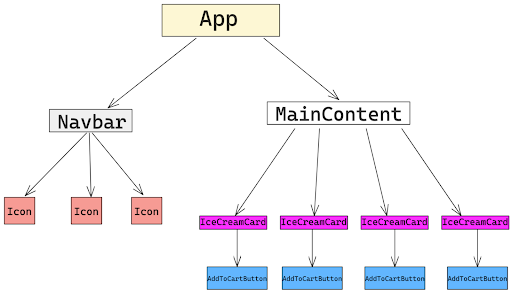
App is the most top level component that acts as a container for the rest of the components. Next are Navbar
and MainContent
as siblings. Navbar
renders those three little icons in the top right of the page. MainContent
renders ice cream cards, which in turn each render a special button.
Let’s look at how we could write this in code without really focusing on the syntax or worrying about specifics. We see that the App
component renders the two siblings (Navbar
and MainContent
), then each of those renders the children for which they are responsible. A great example of the reusability of components happens inside the MainContent
component. We see that for each ice cream, we can use the same IceCreamCard
component. We will pass in slightly different information, but the component itself gets reused for each new ice cream.
Note how in the IceCreamCard
component, we’re actually using plain HTML elements in addition to some custom components. Often, the farther down the tree you go, the more HTML you’ll see as we still use it to create the actual structure of the app we’re building, almost like legos.
const App = () => {
return (
<>
<Navbar />
<MainContent />
</>
);
};
export const Navbar = () => {
const icons = []// some array of icons
return (
{icons.map(icon => <Icon />)}
)
}
export const MainContent = () => {
const iceCreamList = [] // some array of ice creams
return (
{iceCreamList.map(iceCream => (
<IceCreamCard image={iceCream.image} title={iceCream.title} />
))
}
)
}
export const IceCreamCard = ({image, title}) => {
return (
<div>
<img src={image} alt={title}/>
<AddToCartButton />
</div>
)
}
React Disadvantages
We’ve seen how powerful and developer friendly React can be, however there’s no such thing as a perfect solution. React does have some drawbacks as well.
Flexibility (maybe too much?)
The same boon we talked about that allows a developer to create the architecture they would like can also cause a longer development cycle and more opportunities for mistakes as there are more moving parts.
Outdated Documentation
Even though the React community is great, the actual React documentation is quite outdated and not the best source for current best practices.
Steep Learning Curve
Between JSX, component-based architecture, state management and the intricacies of React’s render cycle, learning React can have a steep learning curve — especially for those new to Javascript frameworks or front-end development in general.
React vs. Other Front-End Frameworks
While React is one of the most-used JavaScript libraries for building interfaces, developers have other options, depending on their project requirements. For those looking for alternatives, there are React-based and non-React front-end frameworks. .
React-Based Frameworks
Next.js: Offers server-side rendering, built-in routines and optimization for dynamic web apps.
Gatsby: Optimized for static site generation, making it ideal for content-heavy sites like blogs.
Other Front-End Frameworks
If you’re considering alternatives to React entirely, other prominent front-end frameworks include:
Vue.js: easy-to-learn framework with reactive data binding.
Angular: enterprise-grade framework backed by Google and support for Typescript, routing and state management.
Svelte: compiles components in vanilla JavaScript, leading to faster performance and smaller bundle sizes.
Frequently Asked Questions
What is React used for?
React is used to build dynamic, high-performance user interfaces, allowing updates to content without full page reloads.
How does React improve performance?
React uses a Virtual DOM to batch and optimize updates, reducing the computational cost of direct DOM manipulation.
What is the difference between declarative and imperative programming in React?
Declarative programming lets developers describe what the UI should look like, while imperative programming requires step-by-step instructions to build the UI.
What are components in React?
Components are reusable building blocks that combine HTML and JavaScript, enabling developers to build consistent and maintainable UIs.
What is JSX in React?
JSX is a syntax that allows developers to write HTML-like code directly within JavaScript, making UI structure more intuitive.