Telegram is a messaging app that is similar to WhatsApp and Facebook Messenger. It was created by Russian entrepreneur Pavel Durov and is known for its emphasis on security and speed. Telegram allows users to send messages, photos, videos, and other files to others and to create group chats with up to 200,000 members. It is available on a variety of platforms, including iOS, Android and desktop (Windows and OSX).
Types of Telegram APIs
Telegram offers two kinds of APIs for developers. The Bot API allows you to easily create programs that use Telegram messages for an interface. The Telegram API and TDLib allow you to build your own customized Telegram clients.
In this article, we will be using the Bot API, which allows developers to connect bots to their applications and systems. Bots are special accounts that do not require a telephone number to set up and correspond to an interface for code running on one or multiple servers.
What Is Telegram?
Telegram is a messaging app that is similar to WhatsApp and Facebook Messenger. It was created by Russian entrepreneur Pavel Durov and is known for its emphasis on security and speed. Telegram allows users to send messages, photos, videos, and other files to others and to create group chats with up to 200,000 members. It is available on a variety of platforms, including iOS, Android and desktop (Windows and OSX).
Note that several wrappers exist for the Bot API. These wrappers are written in various languages and let you interact programmatically with the API. For the purpose of this tutorial, we’ll be using the Python client.
Use Cases for Telegram Bot API
Telegram Bot API can be used for a variety of purposes, from video or image manipulation to systems that are responsible for managing notifications. Other potential use cases for Telegram Bot API include building interactive games or providing personalized news updates.
Additionally, the Telegram Bot API allows for the creation of bots that can be easily integrated with other services and interact with external APIs. For example, you could build a notification system that makes use of the Telegram Bot API that, in turn, calls the GitHub Actions API and informs you when a build has failed and/or succeeded.
How to Use the Telegram Bot API
- Install the Python library.
- Get the Telegram bot API token.
- Build the Telegram bot.
1. Install the Python Library
Python-telegram-bot
is an asynchronous interface for the Telegram Bot API. First, let’s create a fresh virtual environment that we will use to install the dependencies required as part of this tutorial:
python3 -m vevn ~/telegram-tutorial-venv
Now that we have created the virtual environment, we can go ahead and activate it:
source ~/telegram-tutorial-venv/bin/activate
And finally, let’s install the Python wrapper for the Telegram Bot API using pip:
pip install python-telegram-bot --pre --upgrade
2. Get the Telegram Bot API Token
In order to make use of the Telegram Bot API, we first need to generate a token that will be used by subsequent requests to the API endpoint (this is going to be handled by the Python client, but we still have to pass this token once).
On your Telegram application (either on your mobile or desktop), search for the BotFather account (make sure to use the verified one):
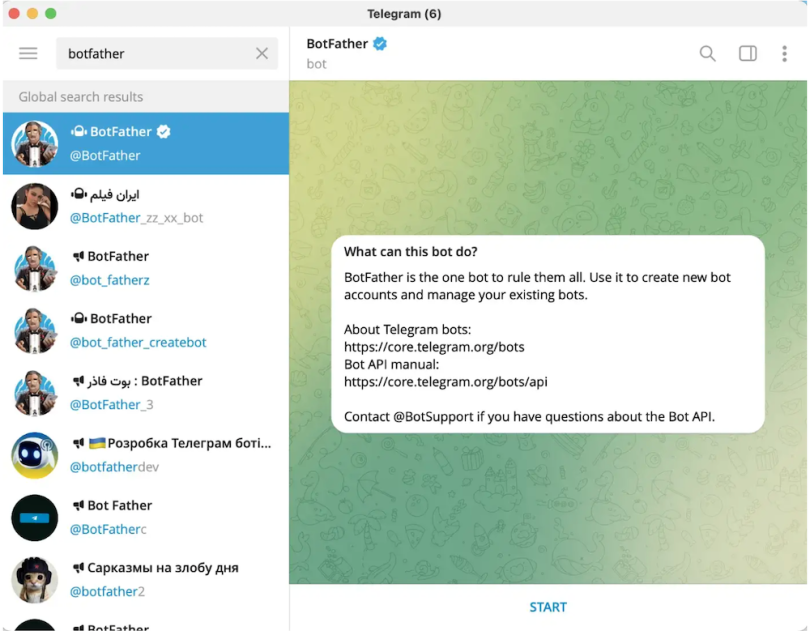
Next, follow these steps:
- Click the Start button on the bottom of the screen.
- Type
/newbot
and click Enter - Then choose a name for the bot.
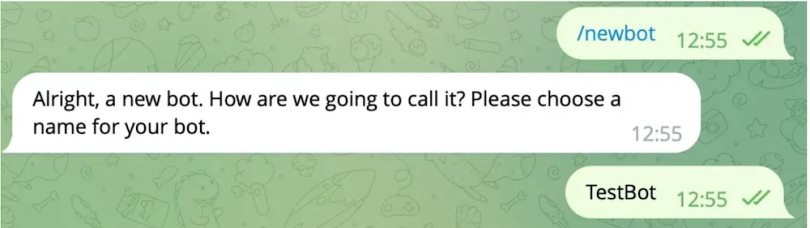
- And, finally, pick a user name (note this must be unique).
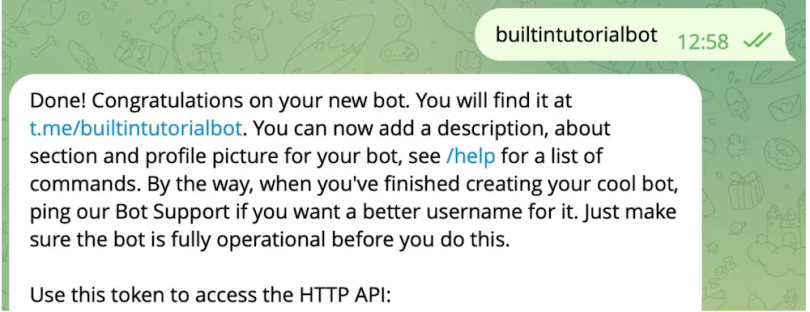
In the response, you should be able to access the token generated, which can be used to control our newly created bot.
3. Build the Telegram Bot
Now that we have generated the token for our newly created Telegram bot, we can go ahead and start building its logic. In order to let users interact with our bot, we need to provide some commands.
First, let’s build a proper welcome message that will be presented to the users whenever they type /start
:
from telegram import Update
from telegram.ext import Application, ContextTypes, CommandHandler
token = '<your-telegram-bot-token>'
async def start(update: Update, context: ContextTypes.DEFAULT_TYPE):
await context.bot.send_message(
chat_id=update.effective_chat.id,
text='Hello and welcome to the BuiltIn Telegram bot!'
)
if __name__ == '__main__':
application = Application.builder().token(token).build()
start_handler = CommandHandler('start', start)
application.add_handler(start_handler)
application.run_polling()
And now, let’s test this functionality:
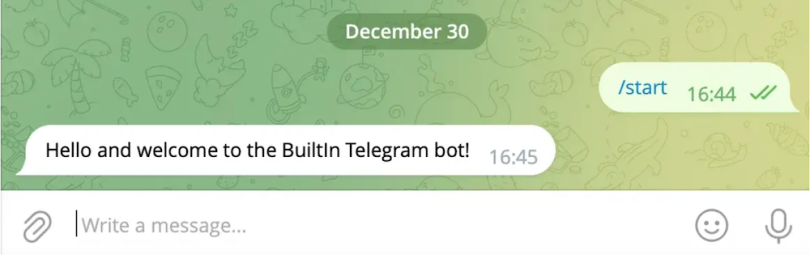
Now, let’s build the /help
command that will essentially list and inform the users about all the commands supported by the bot.
from telegram import Update
from telegram.ext import Application, ContextTypes, CommandHandler
token = '<your-telegram-bot-token>'
async def start(update: Update, context: ContextTypes.DEFAULT_TYPE):
await context.bot.send_message(
chat_id=update.effective_chat.id,
text='Hello and welcome to the BuiltIn Telegram bot!'
)
async def help(update: Update, context: ContextTypes.DEFAULT_TYPE):
await context.bot.send_message(
chat_id=update.effective_chat.id,
text="""
The BuiltIn Telegram bot supports the following commands:
- /start: Welcoming users
- /help: List of supported commands (you are here)
- /first_name: Reports the user's first name
- /last_name: Reports the user's last name
"""
)
if __name__ == '__main__':
application = Application.builder().token(token).build()
start_handler = CommandHandler('start', start)
application.add_handler(start_handler)
help_handler = CommandHandler('help', help)
application.add_handler(help_handler)
application.run_polling()
And here’s the response whenever the user calls the /help
command:
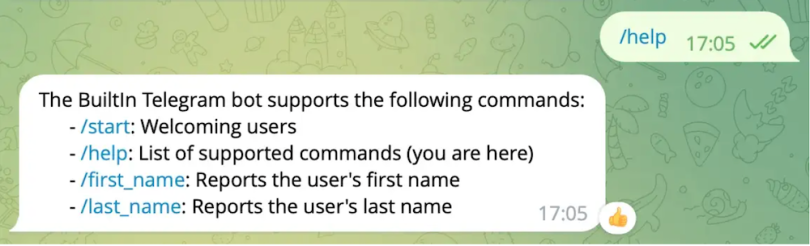
Finally, let’s build two additional handlers that can be used to report users’ first and last names whenever they get called:
from telegram import Update
from telegram.ext import Application, ContextTypes, CommandHandler
token = '<your-telegram-bot-token>'
async def start(update: Update, context: ContextTypes.DEFAULT_TYPE):
await context.bot.send_message(
chat_id=update.effective_chat.id,
text='Hello and welcome to the BuiltIn Telegram bot!'
)
async def help(update: Update, context: ContextTypes.DEFAULT_TYPE):
await context.bot.send_message(
chat_id=update.effective_chat.id,
text="""
The BuiltIn Telegram bot supports the following commands:
- /start: Welcoming users
- /help: List of supported commands (you are here)
- /first_name: Reports the user's first name
- /last_name: Reports the user's last name
"""
)
async def first_name(update: Update, context: ContextTypes.DEFAULT_TYPE) -> int:
await context.bot.send_message(
chat_id=update.effective_chat.id,
text=f'Your first name is {update.message.from_user.first_name}'
)
async def last_name(update: Update, context: ContextTypes.DEFAULT_TYPE) -> int:
await context.bot.send_message(
chat_id=update.effective_chat.id,
text=f'Your last name is {update.message.from_user.last_name}'
)
if __name__ == '__main__':
application = Application.builder().token(token).build()
start_handler = CommandHandler('start', start)
application.add_handler(start_handler)
help_handler = CommandHandler('help', help)
application.add_handler(help_handler)
first_name_handler = CommandHandler('first_name', first_name)
application.add_handler(first_name_handler)
last_name_handler = CommandHandler('last_name', last_name)
application.add_handler(last_name_handler)
application.run_polling()
And here we go — let’s see our finalized bot in action:
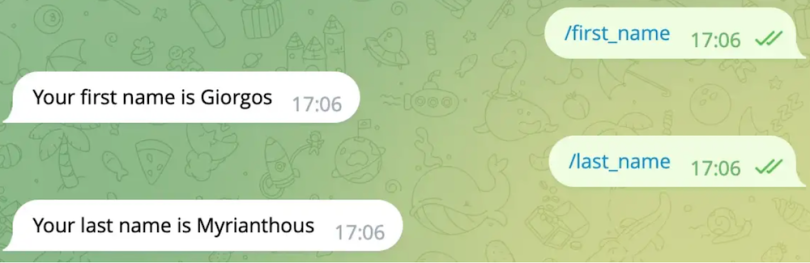
Get Started With the Telegram Bot API
Telegram has been constantly gaining traction and has now become one of the most popular messaging applications around the globe. In this tutorial, we demonstrated how to create a new Telegram bot, generate a token to be used for API calls and how to build functionality around it.
We’ve only touched a very small subset of the Telegram Bot API and the Python client. Make sure to read the documentation for a more comprehensive understanding of the full capabilities of the API and the client itself.