Using the native requestAnimationFrame method in JavaScript, we can make our browser call and repeat an animation function very quickly forever. It calls itself to paint the next frame.
What Is requestAnimationFrame?
RequestAnimationFrame is an animation method in JavaScript that tells the browser to call a specific function and update the animation for the next repaint. It reduces the animation load on a CPU and allows the animations to run more smoothly.
Your callback routine must itself call requestAnimationFrame()
again if you want to animate another frame at the next repaint. requestAnimationFrame()
is one shot. It takes one argument, the callback. Here’s what you need to know.
RequestAnimationFrame Syntax
window.requestAnimationFrame(callback);
callback
is the function called when it’s time to update your animation for the next repaint.
Animations with requestAnimationFrame are non-blocking, which means if you make subsequent calls to requestAnimationFrame, the resulting animations will all occur at the same time.
Advantages of requestAnimationFrame
The goal is for smooth animation to appear at 60 frames per second (fps).
We could write our animation code like this:
setInterval(() => {
// animation code
}, 1000/60);
But using requestAnimationFrame is more effective for two reasons:
- Its animations are smoother because the function is optimized.
- Animations in the inactive tabs will stop running, which allows the CPU to chill.
Let’s see how can we create the above snippet using requestAnimationFrame:
function smoothAnimation() {
// animtion
requestAnimationFrame(smoothAnimation)
}
requestAnimationFrame(smoothAnimation)
How to Use requestAnimationFrame
requestAnimationFrame
also returns an ID you can use to cancel it.
let reqAnimationId;
function smoothAnimation() {
// animtion
reqAnimationId = requestAnimationFrame(smoothAnimation)
}
// to start
function start() {
reqAnimationId = requestAnimationFrame(smoothAnimation)
}
// to end
function end() {
cancelAnimationFrame(reqAnimationId)
}
console.log("reqAnimationId", reqAnimationId)
Check out the demo to see it in action.
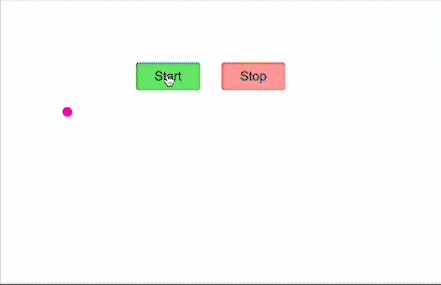
If you do any animation on a browser and want it to be optimized, then I would highly recommend using the requestAnimationFrame web API.