Are you looking for a handy guide for Python to refresh your memory? Look no further. In this article, I cover the most basic features and operations of Python in a condensed format.
This guide should be a great resource for reminding yourself how the syntax of Python works. You may find it particularly useful if it’s been a long time since you last coded in Python.
Comments in Python
You can add comments to your Python code. Single-line comments start with the # symbol. Multiline comments start and end with three apostrophes '''.
#Single line comments start with the '#' -symbol.
'''
Alternatively, you can start and end a multi-line comment with 3 apostrophes
'''
Most Common Data Types of Python
Data types are an important concept in programming. Variables can store different types of data, and these different data types are handled in different ways.
Python has many data types built in by default. I will introduce four of the most common ones here:
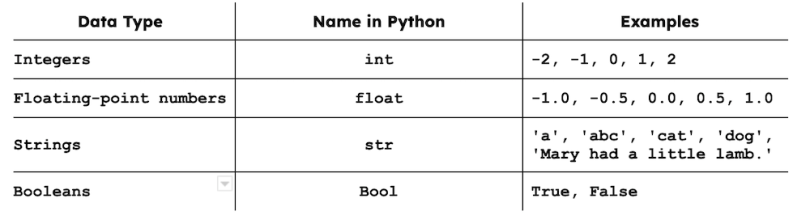
How to Create, Save and Update Variables in Python
In Python, there is no command for declaring variables. Instead, it creates them when you assign a value to them.
No type for a variable is declared either. Instead, Python automatically chooses the data type based on what kind of data you assign to it.
You can create, save and update variables with the assignment operator ‘=’:
#Integer 2 is saved into variable var1:
var1 = 2
#Integer 1 is updated into variable var1:
var1 = 1
#Floating point number 2.0 is saved into variable var2:
var2 = 2.0
#String "Hello, World!" is saved into variable str1:
str1 = "Hello, World!"
#Boolean value True is saved into variable var3:
var3 = True
Naming Variables in Python
In Python, variable names can only contain alphanumeric characters and underscores (A-z, 0-9, and _).
Variable names can’t start with a number.
Variable names are case-sensitive (e.g. a variable named var
is different from a variable named Var
, and these are both different from a variable named VAR
).
#Viable variable names:
var = 1
var2 = 2
VAR_3 = 3
#Viable variable name, that would not overwrite the first one:
Var = 10
#Not viable variable names:
1_var = 1 #Starts with a number
var 2 = 2 #Contains the whitespace character ' ', which is not
#allowed
var-3 = 3 #Contains the dash character '-', which is not allowed
'''
Viable but not often used variable name (the underscore character '_' in front of the name signifies that the variable, function or method should only be used internally):
'''
_var = 1
Type Casting in Python
You can also cast data into a different type than the default one:
#Boolean value True is saved into variable var:
var = True
#var is updated to the string 'True' by casting:
var = str(True)
Python Math Operators
Here is a list of math operators in Python in the order of highest to lowest precedence:
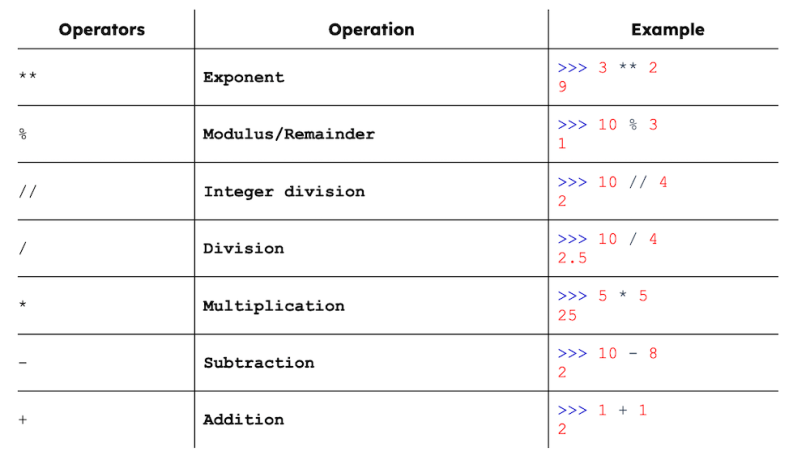
Augmented Assignment Operators in Python
On top of the usual assignment operator =, Python also has augmented assignment operators, which chain math operators with the assignment operator:
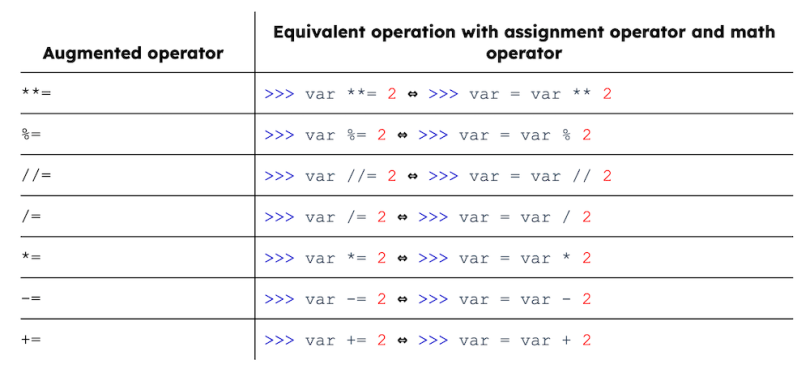
Comparison Operators in Python
Here is a list of comparison operators in Python:
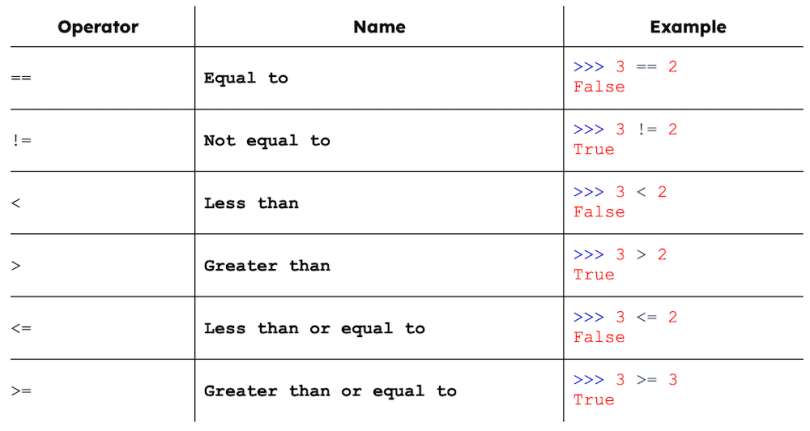
You can use these to compare different kinds of data. These evaluate True
or False
depending on the values you input.
Boolean Operators in Python
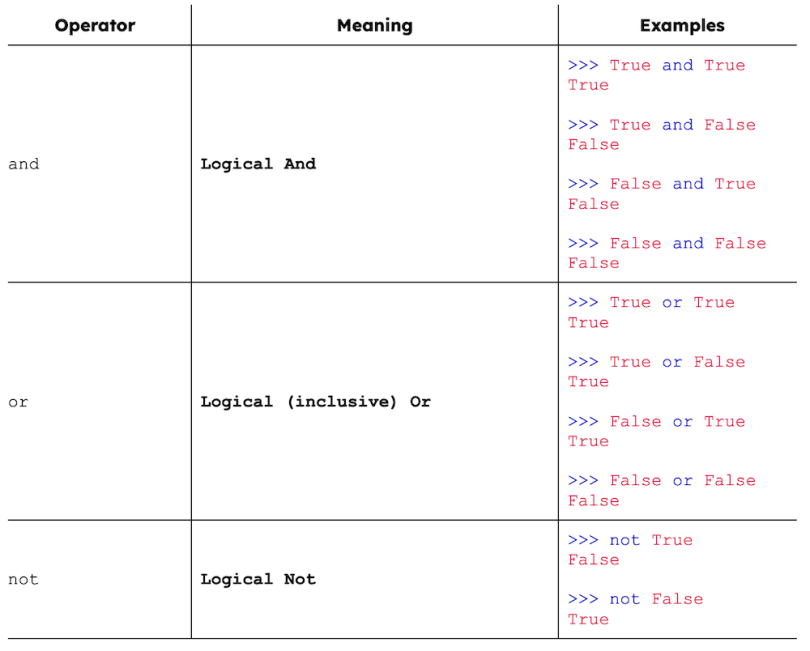
Strings and String Operations in Python
We already saw some examples of strings earlier, but let’s now take a deeper look.
In Python, strings start and end either with the single quotation mark ', or the double quotation mark ". Multiline strings can be assigned to variables by using three quotation marks.
#This is how you assign a string to a variable:
str1 = 'Hello, World!'
str2 = "Hello, World!"
#Strings str1 and str2 are equivalent.
#This is how you assign a multiline string to a variable (single quotations would work as well):
long_str = """Lorem ipsum dolor sit amet,
consectetur adipiscing elit,
sed do eiusmod tempor incididunt
ut labore et dolore magna aliqua."""
Strings in Python are actually arrays of bytes that represent Unicode characters. Square brackets can be used to access elements of the string. This is called string indexing:
>>> "Hello, World!"[0]
'H'
String slicing is similar to string indexing, except then you select a larger portion of the string:
>>> "Hello, World!"[0:5]
'Hello'
The in
operator can be used to check whether a substring is contained in a string (it can be generally used to check if a value is present in a sequence):
>>> "Hello" in "Hello, World!"
True
>>> "HELLO" in "Hello, World!"
False
The not
boolean operator can be chained with the in
operator:
>>> "Hello" not in "Hello, World!"
False
You can use the upper()
and lower()
methods to transform strings into upper- and lower case. You can use the isupper()
and islower()
methods to check whether a string is in upper or lower case:
>>> "Hello".upper()
'HELLO'
>>> "Hello".lower()
'hello'
>>> "Hello".islower()
False
>>> "HELLO".isupper()
True
You can use the + operator to concatenate (or join) two strings:
>>> "Hello" + ", World!"
'Hello, World!'
Use the join()
method to join multiple strings (and specify the separator), the split()
method to split strings, and the replace()
method to replace a part of a string with another string:
>>> ", ".join(["Hello", "World!"])
'Hello, World!'
>>> #The default separator of the split() method is whitespace:
>>> "Hello, World!".split()
['Hello,', 'World!']
>>> #But you can also define the separator yourself:
>>> "Hello, World!".split("l")
['He', '', 'o, Wor', 'd!']
>>> #Use the replace() method to replace with a specific part of a
>>> #string with something else:
>>> "Hello, World!".replace("H", "G")
'Gello, World!'
When you want to format strings in Python, use the format()
method. Formatting means adding numbers to the string. This method takes an unlimited number of arguments and places them into curly bracket placeholders {}
in the string:
>>> name = "John"
>>> amount = 10
>>> food_item = "apple"
>>> "{} ate {} {}s.".format(name, amount, food_item)
'John ate 10 apples.'
You can check the length of a string in characters with the len()
method (which is actually a method generally for iterables):
>>> len("Hello, World!")
13
Conditional Statements in Python
Like many other programming languages, Python supports conditional statements if
, elif
and else
that make use of the comparison operators introduced above. The comparison statements can also be chained together with the boolean operators, which are also introduced above.
After the logical statement, Pythons syntax requires you to use a semicolon (:), after which typically comes a line break and indentation. Indentation (white space at the beginning of a line) is used in Python to define the scope of the code (rather than curly brackets ‘{}
’ like in many other programming languages).
Here are a couple of handy examples of how to use conditional statements in Python:
a = 1
b = 2
#Print something, if a is greater than b:
if a > b:
print("a is greater than b")
#Print something, if a is greater than b, and something else if not:
if a > b:
print("a is greater than b")
else:
print("a is not greater than b")
#Print something, if a is greater than b, something else if a is equal to b and something else if not:
if a > b:
print("a is greater than b")
elif a == b:
print("a is equal to b")
else:
print("b is greater than a")
Using the and
boolean operator to chain two logical statements:
#Print something, if a is greater than b and b is equal to c:
c = 3
if a > b and b == c:
print("a is greater than b, and b is equal to c")
else:
print("a is not greater than b, and/or b is not equal to c")
Chaining multiple conditional statements is possible as well. This is also known as nested conditional statements:
c = 3
if a > b:
if b == c:
print("a is greater than b, and b is equal to c")
else:
print("a is greater than b, but b is not equal to c")
else:
print("a is not greater than b")
You can also use short-hand one line expressions for if
and if
… else
statements. Here are a couple of examples:
#One line if:
if a > b: print("a is greater than b")
#One line if … else:
print("a is greater than b") if a > b else print("a is not greater than b")
Python does not allow empty conditional statements, but if you need one for whatever reason, you can use a pass
statement to avoid getting an error:
#Does not produce an error:
if 1 > 2:
pass
Lists in Python
You can use lists in Python to store multiple items (with possibly different data types) in a single variable. Tuples are defined with square brackets []
. A list in Python is ordered, changeable, and allows for duplicate members. Here are examples of using a list:
#Defining a list and printing it:
my_list = [1, 2, "Hello, World!"]
print(my_list)
#Indexing of lists starts from 0, and you can access elements of the
#list with square brackets:
print(my_list[0]) #Print the first element of the list
print(my_list[-1]) #Print the last element of the list
#Updating an element in the list:
my_list[2] = 3 #The last element is now 3
print(my_list)
#You can append items to the end of the list with the append()
#method, or insert items at a specific index with the insert()
#method:
my_list.append(4) #Appends 4 to the end of the list
my_list.insert(0,"Hello, World!") #Inserts a string to index 0
#in the list
print(my_list)
#You can remove specific items from the list with the remove()
#method, or remove items at a specific index with the pop() method:
my_list.remove(4) #Removes 4 from the list
my_list.pop(0) #Removes the first item in the list
print(my_list)
#You can get the length of the list with the len() method:
print(len(my_list))
#You can concatenate lists with the + operator or with the extend
#method:
print(my_list + [5, 6, 7]) #Makes a new list
my_list.extend([5, 6, 7]) #Adds new elements to existing list
print(my_list)
Tuples in Python
You can use tuples in Python to store multiple items, possibly with different data types) in a single variable. Tuples are defined with round brackets ()
. A tuple differs from a list in that it is unchangeable (meaning you cannot change it, add items to it or remove items from it after it has been created). Here is an example of using a tuple:
#Defining a tuple
tuple_var = (1, 2, "Hello, World!")
#Indexing is done with the square brackets []. The first item has index
#0. This line can be used to access the last element in the above
#tuple:
tuple_var[2]
Dictionaries in Python
You can use dictionaries in Python to store key-value pairs. Dictionaries can have different data types and are defined with curly brackets {}
. Keys and values are separated with the colon and separate elements are separated with the comma. Dictionaries are ordered, changeable, and do not allow duplicates. Here’s an example of using a dictionary:
#Defining a dictionary
dict = {
"name": "John",
"age": 42,
"favorite color": "Blue"
}
#Accessing values is done with the square brackets []. An example of
#accessing values based on the keys:
info_str = "{} is {} years old and their favorite color is {}.".format(dict["name"], dict["age"], dict["favorite color"])
print(info_str)
Sets in Python
You can use sets in Python to store multiple items, possibly with different data types, in a single variable. Tuples are defined with curly brackets {}
. A set is unordered, unchangeable (however, you can add and delete items), and unindexed. Here is an example of using a set:
#Defining a set
new_set = {"Blue", "Green", "Red", 1, 2}
#Since sets are unordered, you can’t know the order in which items
#will be printed. Here’s how you print a set:
print(new_set)
#Here’s how you add and remove items:
new_set.add("Yellow")
new_set.remove(1)
new_set.remove(2)
print(new_set)
Loops in Python
Python supports for loops and while loops.
You can execute a set of statements as long as a condition is true using the while
loop. The syntax for a while
loop starts with the while
-statement, followed by the condition, and then by a colon. The set of statements to be executed in the loop follow this first line and are indented. The indentation defines the scope of the loop, similarly to conditional statements introduced earlier. Here are a couple examples of while loops:
#This loop prints numbers from 1 to 9
i = 1
while i < 10:
print("i is currently {}".format(i))
i += 1
#You can use nested while loops:
i = 1
j = 1
while i < 10:
while j < 10:
print("i is currently {}, and j is {}".format(i,j))
j += 1
i += 1
You can use a for
loop to iterate over a sequence, like a list, tuple, dictionary, set, or a string. The syntax is similar to a while loop, except you replace the while
statement with a for
statement, and the condition with an iterator variable, an in
statement, and a sequence. Here are a couple of examples using the for loop:
#This for loop prints the characters in the string "Hello, World!"
for char in "Hello, World!":
print(char)
#Using the range() -function in a for loop to print numbers from 0
#to 9
for i in range(10):
print(i)
#Iterating (or looping) through a list:
for x in ["Hello, World!", 1, 2, 3]:
print(x)
#And here is an example of two nested for loops:
for i in range(10):
for j in range(10):
print("i is currently {}, and j is {}".format(i,j))
You can also use the continue
, break
, pass
, and else
statements in loops.
You can use the break
statement to stop the execution of the loop before the condition of a while loop is met or before all items of a for loop have been iterated over:
#These loops print numbers from 0 to 4, because they stop when they
#reach i == 5
i = 0
while i < 10:
if i == 5:
break
print(i)
i += 1
for i in range(10):
if i == 5:
break
print(i)
You can use the continue
statement to stop the current iteration of a loop and continue with the next iteration:
#These loops print numbers from 0 to 9, except they skip printing
#number 5
i = 0
while i < 10:
if i == 5:
continue
print(i)
i += 1
for i in range(10):
if i == 5:
continue
print(i)
Python does not allow empty for loops, but if you need one for whatever reason, you can use a pass statement to avoid getting an error:
#This loop does nothing sensible but does not produce an error
for i in range(10):
pass
You can also use an else
statement to execute a set of statements when a loop is finished:
#These loops print the numbers from 0 to 9, and when they are finished, they print "Loop is finished"
i = 0
while i < 10:
print(i)
i += 1
else:
print("Loop is finished")
for i in range(10):
print(i)
else:
print("Loop is finished")
Built-In Python Functions
We have already seen some built-in Python functions, but let’s go through some of the most common and generally useful ones.
print()
The print()
function can be used to print objects (most often strings) to the text stream file. Example:
>>> print("Hello, World!")
Hello, World!
range()
The range()
function returns a sequence of numbers. By default, it starts from zero and uses increments of one, but you can specify these to your liking. The only argument you need to give is the number before which it stops. The syntax is range(start, stop, step)
. This function is useful when building for loops, among other things, and I will demonstrate its use in this context. Example:
>>> #Starts from 0, increments by 1 and stops before 4:
>>> for i in range(4): print(i)
0
1
2
3
>>> #Starts from 2, increments by 3 and stops before 10:
>>> for i in range(2,10,3): print(i)
2
5
8
len()
The len()
function returns the length, or number of items of an object. Example:
>>> #Length of a string:
>>> len("Hello, World!")
13
>>> #Length of a list
>>> len([1, 10, 3, "Hello, World!"])
4
max()
The max()
function returns the largest item of an iterable. Example:
>>> #Max of a list:
>>> max([1,2,5,1000])
1000
min()
The min()
function returns the smallest item of an iterable. Example:
>>> #Min of a list:
>>> min([1,2,5,1000])
1
sum()
The sum()
function returns the sum of elements in an iterable. You can also specify a start value, to which the sum()
function starts to add. Example:
>>> #Sum of a list:
>>> sum([1,2,5,1000])
1008
>>> #Sum of a list, starting from 1000:
>>> sum([1,2,5,1000],1000)
2008
input()
The input()
function allows a Python program to take text input from the user. When the function is executed, the program stops to wait for input from the user. Example (note that in the example, the user needs to type “James Bond”):
>>> print("What is the name of your character?")
What is the name of your character?
>>> char_name = input()
James Bond
>>> print("Hello {}!".format(char_name))
Hello James Bond!
>>> #You can also specify a default message in the input() function:
>>> char_name = input("What is the name of your character?\nName: ")
What is the name of your character?
Name: James Bond
>>> print("Hello {}!".format(char_name))
Hello James Bond!
Function Definition in Python
Use functions in Python to execute a set of statements when calling the function. Functions can take arguments (also known as parameters) and return data as well.
Functions are defined with the def
keyword, followed by a colon. After this initial line, use white space to define the scope of the function.
Here’s an example of a simple function that takes two numbers as arguments and returns their sum:
def sum_of_two_numbers(num1, num2):
return num1 + num2
print(sum_of_two_numbers(5,10))
Keyword arguments and default parameter values are also useful concepts when dealing with functions.
You can use keyword arguments to send arguments with the key = value
syntax. The point is that the order of the arguments does not matter when doing this.
With the default parameter value, you can define a default value for a parameter that is used when the function is called without that argument.
Here’s an example demonstrating the use of keyword arguments and default parameter values:
def my_fun(name, age, favorite_color = "Blue"):
info_str = "{} is {} years old and their favorite color is {}.".format(name, age, favorite_color)
print(info_str)
#Not using keyword arguments or the default parameter value:
my_fun("John", 42, "Red")
#Using keyword arguments and the default parameter value:
my_fun(age = 52, name= "John")
Classes in Python
Python is an object-oriented programming language, so it’s only natural it also has classes. Classes are an object constructor, so you can define them to your liking.
To create a class, use the class
keyword followed by a colon : . After this line, use indentation to define the scope of the class and add the properties you wish.
You can use the __init__()
function to define initiation for the class. Use this function to assign necessary values to the class properties and do other necessary operations for creating an instance of the class (or, in other words, creating an object).
You can use the __str__()
function to define what is returned when the object is represented as a string.
Use the self
parameter to reference the current instance of the class. It has to be the first parameter in any function used in the class.
Here is an example of creating and using a class:
class human:
def __init__(self, name, age, favorite_color):
self.name = name
self.age = age
self.favorite_color = favorite_color
def __str__(self):
info_str = "{} is {} years old and their favorite color is {}.".format(self.name,self.age,self.favorite_color)
return info_str
new_human = human("John", 42, "Blue")
print(new_human)
#You can modify object properties:
new_human.age = 52
print(new_human)
#You can also delete object properties:
del new_human.age
print(new_human) #This will now produce an error
#You can also delete objects:
del new_human
print(new_human) #This will now produce a different error