Python’s flexibility makes it the best solution to automate and to do repetitive tasks in an efficient way.
In Python (and other programming languages), loops help to iterate over a list, tuple, string, dictionary and a set.
Pass vs. Continue in Python Explained
- Pass: The pass statement in Python is used when a statement or a condition is required to be present in the program, but we don’t want any command or code to execute. It’s typically used as a placeholder for future code.
- Continue: The continue statement in Python is used to skip the remaining code inside a loop for the current iteration only
- Break: A break statement in Python alters the flow of a loop by terminating it once a specified condition is met.
Loops iterate over a block of code until the test expression is false, but sometimes we wish to terminate the current iteration or even the whole loop without checking the test expression.
This can be achieved using a few keywords that can alter the flow or execution of the loops. In Python those keywords are — break, continue and pass. It’s important to know when and how to use these keywords to control the flow of your loop.
Therefore, I’ll discuss and provide examples for the when, why and how of break, continue and pass in Python. The concept behind these keywords is the same regardless of the programming language you choose to use.
Let’s get started.
Break Statement in Python
The break statement in Python terminates the loop containing it.
For example:
for num in range(0,10):
if num == 5:
break
print(f'Iteration: {num}')
The above for loop generates output as:
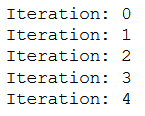
We’ve added an if
statement to check a condition. When this condition becomes True
, the program flow will go inside the if
statement and execute the break
statement.
Therefore, the for
loop executed until the condition num == 5
becomes True
. When the condition becomes True
, the break
statement is executed to terminate the flow of the for
loop.
Now, let’s add one more for
loop outside the existing for
loop, as shown below:
for k in range(0,5):
print(f'Outer For Loop Iteration: {k}')
for num in range(0,10):
if num == 5:
break
print(f'--Inner For Loop Iteration: {num}')
The break statement will terminate the for loop containing it when the condition becomes True.
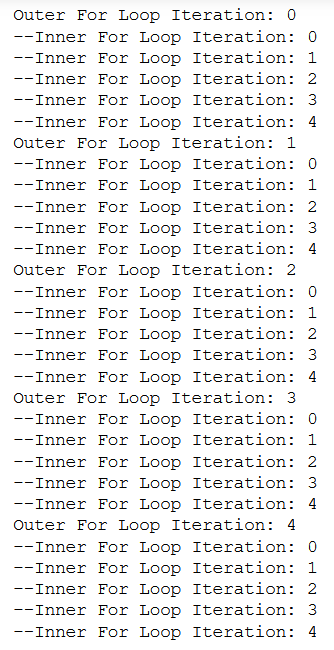
As we can see in the above picture, for every iteration of the outer for loop, the flow of the inner loop breaks after five iterations, as per the condition num == 5
.
Thus, if the break
statement is inside a nested loop (a loop inside another loop), the break
statement will terminate the innermost loop.
A real world example would be unlocking our smartphone with a password. Technically, the phone unlocking process is a for
loop that continually asks for the password. When a correct password is entered, a break
statement gets executed and the for
loop gets terminated.
Below is the explanation with code:
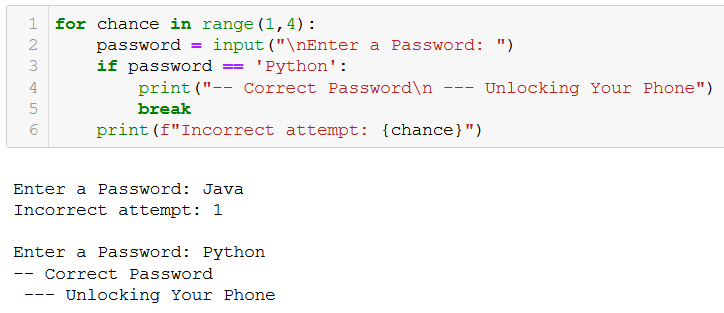
In the above example, I set Python as the correct password. When the condition password == ‘Python’
becomes True, a break statement is executed and the for loop gets terminated.
Pass Statement in Python
As its name suggests, the pass
statement does nothing.
It’s used when a statement or a condition is required to be present in the program, but we do not want any command or code to execute.
For instance, let’s replace the break
statement in the previous example with a pass
statement.
for num in range(0,10):
if num == 5:
pass
print(f'Iteration: {num}')

As we see in the above output, the pass statement really did nothing as the for loop and all statements within it are executed.
The pass statement is typically used while creating a method that we don’t want to use right now. It’s often used as a placeholder for future code.
Most of the time, a pass statement is replaced with another meaningful command or code in a program.
Continue Statement in Python
The continue
statement is used to skip the remaining code inside a loop for the current iteration only.
For instance, let’s use continue
instead of a break
statement in the previous example.
for num in range(0,10):
if num == 5:
continue
print(f'Iteration: {num}')
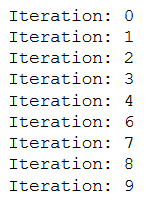
When the condition num == 5
becomes True
, the continue
statement gets executed. The remaining code in the loop is skipped only for that iteration. That’s why Iteration: 5
is missing from the above output.
Therefore, the continue
statement works opposite to the break
statement. Instead of terminating the loop, it forces it to execute the next iteration of the loop.
Pass vs. Continue in Python
There is a significant difference between pass and continue, and they are not interchangeable.
Continue
forces the loop to start at the next iteration, whereas pass
means, “there is no code to execute here,” and it will continue through the remainder of the loop body.
Differences Between Pass and Continue in Python
Pass and continue statements are not interchangeable in Python. A pass statement signals to a loop that there is “no code to execute here.” It’s a placeholder for future code. A continue statement is used to force the loop to skip the remaining code and start the next iteration.
Here is an quick explanation of this difference:
for num in range(0,5):
pass
print(f'Iteration: {num}')
print("This statement is after 'pass'")
continue
print("This statement is after 'continue'")
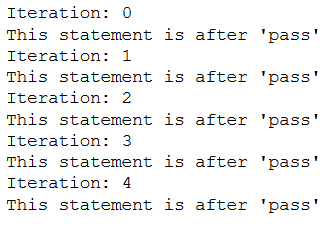
In the above output, in each iteration of the for
loop, the first two print statements are always executed even though they are after the pass
statement. This means pass
does nothing, and the flow of the loop goes un-interrupted.
However, the print statement after the continue
statement isn’t executed, as the keyword continue
forced the for
loop to start the next iteration.
Using Pass, Continue and Break in Python
Summing up, a single example would be more helpful for understanding how pass, continue and break work in Python.
number_of_iterations = int(input("How many iterations you want ? \n "))
for iteration_count in range(1,1000):
print(f"\nInteration: {iteration_count}")
print("\nThis is line before 'pass'")
pass
print("\nThis is line after 'pass'")
if iteration_count < number_of_iterations:
print("\nThis is line before 'continue'")
continue
print("\nThis is line after 'continue'")
print("\nThis is line after condition check for 'continue'")
if iteration_count < number_of_iterations+1:
print("\nThis is line before 'break'")
break
print("\nThis is line after 'break'")
print("\nThis is line after condition check for 'break'")
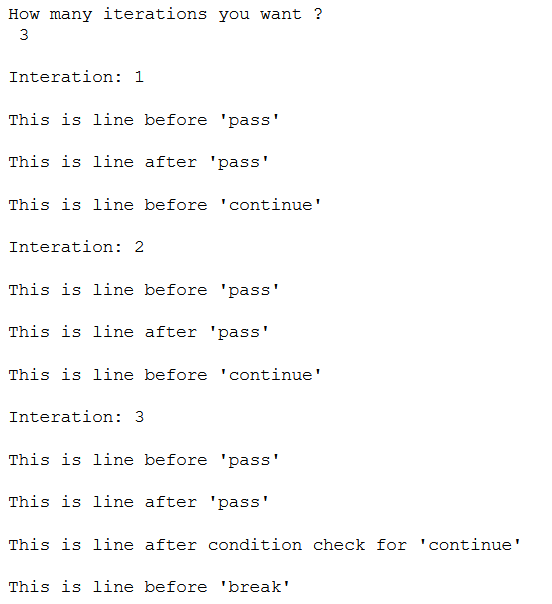
As you can see in the above output, everything before and after pass
is always executed, indicating the keyword pass
does nothing.
Only the line before continue
keyword is executed, indicating that continue
always forces the for
loop to start the next iteration and doesn’t execute anything after the keyword continue
in the current iteration.
Finally, the line before break
gets executed and the flow of the for loop gets terminated on the third iteration. This explains how the break
statement is used to stop the process.
Frequently Asked Questions
When should you use pass in Python?
Pass
in Python is used as a placeholder for future code in statement blocks and loops. Nothing happens when a pass statement is executed, but it can fill statement spaces in if-else statements, for
loops and while
loops so no errors are returned when code is run.
What is the difference between break and continue in Python?
In Python, break
stops a loop once its condition is met, while continue
skips over the current iteration of a loop and continues to the next iteration based on its condition.