The Foobar challenge is a coding test hosted by Google that can be completed in either Python or Java. I completed the challenge using Python. The challenge has its own server with specific, terminal-style commands.
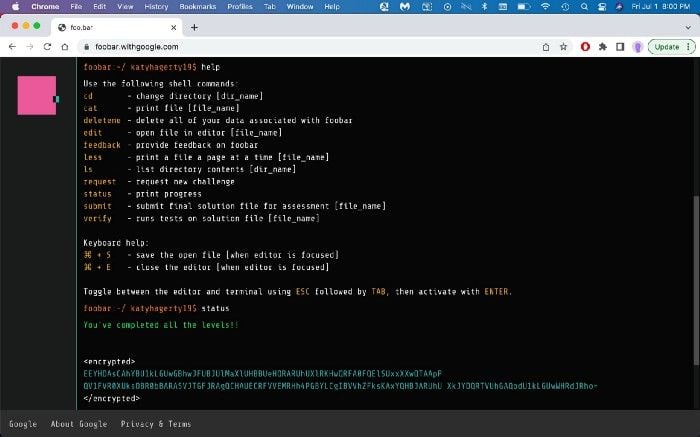
To add some fun, it’s space themed. The evil Commander Lambda has kidnapped space bunnies, and you must rescue them. Each problem adds more context to the backstory. The problems are of various difficulty and are organized into five levels. Each question must be solved within a certain time limit. More time is given for the higher levels.
What Is the Google Foobar Challenge?
The Google Foobar challenge is a coding test administered by Google that consists of five levels and can be completed in either Python or Java. The challenge has been used to evaluate and recruit potential engineers for Google. There are two ways to participate: Google extends an invitation over the browser or you receive an invitation code.
I found the levels one, two and three problems similar to LeetCode problems, both in terms of difficulty and structure. The problems were straightforward and rarely required optimizing code for speed. On the other hand, levels four and five were more complicated and layered multiple concepts into one problem.
How to Participate in the Foobar Challenge?
There are two ways to participate in the challenge:
- Google extends an invitation through the browser.
- A friend sends you an invitation code.
I came across the challenge through the first method. I was Googling “list comprehension” for a different article, and my browser unfolded to reveal the invitation. I had never heard of the Foobar challenge before and was a little circumspect. After verifying that this was a legitimate challenge, I accepted.
Needless to say, the element of surprise piqued my interest in the challenge. Not to mention, Google reaching out to me with an exclusive invite definitely gave me a confidence boost as a developer. I saw this as a call to adventure and immediately wanted to devote all my attention to it.
For the second method, you need a friend to send you an invitation code. One invitation code is given after completing level two. Another is given after completing level four.
Does Google Use the Challenge for Recruiting?
At one point, Google used the challenge to find new talent. You’ll find many people online who had a Google recruiter reach out one or two days after completing the challenge. However, it appears that Google hasn’t used the challenge for hiring since 2020.
After finishing level three, the Foobar server asks if you want to provide your contact info to a Google recruiter. I provided my info but never heard from them.
Nevertheless, I still believe this challenge is worthwhile. It will expose you to new coding concepts, sharpen your skills, and ultimately, make you a better developer.
Google Foobar Tips
Google provides a constraints.txt
file, but it isn’t very detailed. For instance, it states all standard libraries are allowed with a few listed exceptions. However, math
and numpy
aren’t allowed even though those are common libraries. To test which libraries were allowed, I imported the library, returned the answer of one of the test cases and then verified the solution. If the solution passed the one test case, the library was acceptable.
# Trick to test if library is allowed
import numpy as np
def solution(n):
return 1
Also, comment out all print statements before verifying the code. Any print statements will cause the test cases to fail.
The challenge is very particular about input and output types. If the problem statement asks for a specific input or output type, make sure to supply the correct type.
Lastly, I didn’t have any experience with Python 2.7 prior to this challenge. In fact, I tested many of my solutions for the lower level problems in Python 3. This came back to bite me later in the challenge. Specifically, I was not aware that in Python 2.7.13, / operator performs integer division if the inputs are integers. For instance:
# Integer division in Python 2.7.13
a = 5
b = 2
print(a / b)
>>>2
However, in Python 3, / performs float division.
# Float division in Python 3
a = 5
b = 2
print(5 / 2)
>>>2.5
Google Foobar Questions and Concepts
Below, I breakdown the questions and explain my thought process. I also provide solutions. However, I highly recommend you attempt the problem first. The best part of the challenge is the surprise and satisfaction of solving an elusive problem.
I debated not posting solutions and just explaining the underlying concepts. However, part of coding is learning how to troubleshoot and pinpointing where the code fails. Thus, I decided to post solutions so that if you’re stuck, you can see exactly where your logic diverges.
Google Foobar Level 1: Problem 1
The Set Up
I Love Lance & Janice
“You’ve caught two of your fellow minions passing coded notes back and forth — while they’re on duty, no less! Worse, you’re pretty sure it’s not job-related — they’re both huge fans of the space soap opera “”Lance & Janice””. You know how much Commander Lambda hates waste, so if you can prove that these minions are wasting her time passing non-job-related notes, it’ll put you that much closer to a promotion.
Fortunately for you, the minions aren’t exactly advanced cryptographers. In their code, every lowercase letter [a..z] is replaced with the corresponding one in [z..a], while every other character (including uppercase letters and punctuation) is left untouched. That is, ‘a’ becomes ‘z’, ‘b’ becomes ‘y’, ‘c’ becomes ‘x’, etc. For instance, the word “”vmxibkgrlm””, when decoded, would become “”encryption””.
Write a function called solution(s) which takes in a string and returns the deciphered string so you can show the commander proof that these minions are talking about “”Lance & Janice”” instead of doing their jobs.”
Languages
- To provide a Python solution, edit
solution.py
. - To provide a Java solution, edit
solution.java
.
Test Cases
Inputs:
(string) s = “wrw blf hvv ozhg mrtsg’h vkrhlwv?”
Output:
(string) “did you see last night’s episode?”
Inputs:
(string) s = “Yvzs! I xzm’g yvorvev Lzmxv olhg srh qly zg gsv xlolmb!!”
Output:
(string) “Yeah! I can’t believe Lance lost his job at the colony!!”
Use verify [file]
to test your solution and see how it does. When you are finished editing your code, use submit [file]
to submit your answer. If your solution passes the test cases, it will be removed from your home folder.
How to Solve Google Foobar Level 1
Coincidentally, I recently built an app for a shift cipher and this problem immediately reminded me of that. Only the lowercase characters need to be deciphered. The other characters remain unchanged. First, create a dictionary to store the encoded characters and their deciphered counterparts. Next, use a for loop to iterate through every character in the input string. If the character is lowercase, pull its deciphered value from the dictionary. If not, add the character to the answer string.
In my opinion, this problem was designed to test the participant’s knowledge of the str
and dict
data types and their built-in methods.