In C++, you might often come across the line “using namespace std;”
. The line signals to the compiler to treat all names in the std namespace
as if they’re in the global namespace.
“Using Namespace STD;” in C++ Explained
The line “using namespace std;
” in C++ signals to the compiler to treat all names in the std namespace
as if they’re in the global namespace. This means you can use names without specifying std::
before them. It’s considered bad practice because it can create naming conflicts, decrease code readability and make it difficult to update code without future naming conflicts.
It seems convenient, but it’s often discouraged in professional coding practices. In this guide, we’ll explore why this seemingly innocent line can lead to issues and what better alternatives exist.
What Is “Using Namespace STD;” in C++?
The std namespace
in C++ contains many standard library components, including data types, functions and objects. When you write “using namespace std;”
, you’re essentially telling the compiler to consider all the names in the std namespace as if they’re in the global namespace. This means you can use names like cin
, cout
and vector
without specifying std:: before them.
Disadvantages of “Using Namespace STD;” in C++
1. Namespace Pollution
When you bring all of std into the global namespace, you risk naming conflicts. If your code or any library you’re using defines a name that clashes with something in std, you'll have a problem.
2. Readability
In larger projects, it can become unclear where a particular name is coming from. This can make code harder to understand and maintain.
3. Maintenance Challenges
If you later need to add a new name to your code that happens to exist in std
, you’ll have to refactor your entire codebase to resolve naming conflicts.
Why You Should Use Explicit Namespace in C++
Instead of bringing the entire std namespace into your code, consider using specific names from std explicitly.
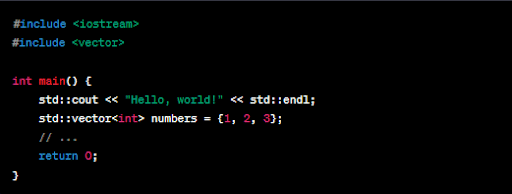
Benefits of Explicit Namespace Usage
- Clarity: It’s clear where each name is coming from, making your code more readable.
- Avoiding Conflicts: You won’t run into conflicts with names defined elsewhere in your code or in libraries.
- Maintainability: It’s easier to maintain your code in the long term, especially in larger projects.
While using “using namespace std;”
might seem convenient, it’s generally considered bad practice due to the potential for naming conflicts and reduced code readability. Embracing explicit namespace usage is a better approach, ensuring your code remains clean, maintainable and free of unexpected issues.
Frequently Asked Questions
What is namespace std in C++?
In C++, std namespace
contains the names and identifiers of standard library components, like data types, functions and objects for a project. It separates these names from others that are in the global namespace.
What is the problem with “Using Namespace STD;” in C++?
There are three issues with using “using namespace std;”
. It can increase the risk of naming conflicts with other names in the global namespace, it makes the code more difficult to read and it can make it more difficult to add new names without having to refactor old code. It’s considered best practice to use explicit names from the namespace exclusively.