HashMap is a data structure that implements the Map interface and uses a hash table for storing key-value pairs. It allows the insertion of elements based on key-value pairs and provides constant-time performance for basic operations like adding or retrieving elements.
HashMap in Java Explained
HashMap is a data structure that uses the Map interface and a hash table for storing key-value pairs. It’s a widely used data structure in Java that provides efficient access and manipulation of data based on unique keys.
What Is HashMap in Java?
HashMaps are a versatile and widely used data structure in Java, offering efficient access and manipulation of data based on unique keys.
Here are some key points about HashMaps in Java:
- Key-Value Pairs: HashMap stores elements as key-value pairs where each key is unique. Keys are used to retrieve corresponding values.
- Fast Retrieval: Retrieving elements from a HashMap by their keys is efficient because it calculates the hash code of the key and directly fetches the corresponding value from the computed index.
- Null Keys and Values: HashMap allows a single null key and multiple null values. This means one key can be null, but duplicate keys are not permitted, as keys must be unique.
- Hashing Mechanism: Internally, HashMap uses hashing to determine the storage location for elements. It applies a hash function to keys to compute their hash codes, converting these codes into array indices where the elements are stored.
- Iteration: Iterating through the elements of a HashMap is possible using iterators or enhanced for loops.
When to Use HashMap in Java?
Because of its effective retrieval capabilities and key-value pair storing technique, Java’s HashMap is a flexible data structure that may be used in a variety of contexts.
It enables rapid access to elements based on their keys, regardless of their position within the collection. This flexibility makes it a cornerstone for various applications, from managing user information to creating dynamic lookup tables.
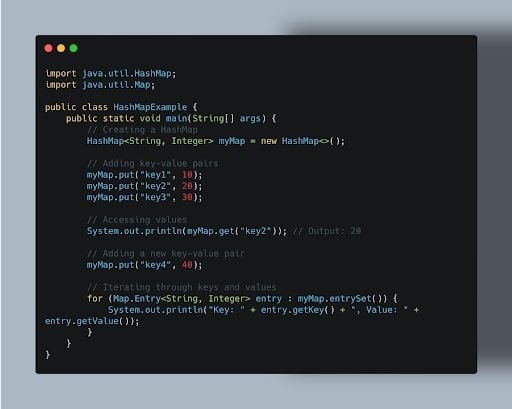
Here are some scenarios in which a HashMap could be useful:
Fast Retrieval of Data
When you need to store data where quick access to values based on unique keys is essential, HashMap provides efficient retrieval with a time complexity of O(1) on average for get()
and put()
operations. This makes it suitable for applications requiring fast access to key-value pairs.
Implementing Caches
HashMaps can be utilized to implement simple caching mechanisms where frequently accessed data is stored temporarily. This avoids redundant computations or database calls improving code performance.
Unique Key-Value Storage
If you need to store unique keys with associated values, such as a dictionary where words are keys and their meanings are values, HashMap ensures that the keys are unique. This uniqueness allows easy retrieval and update of specific values based on their corresponding keys.
Mapping Relationships
HashMaps are ideal for establishing relationships between different entities. For example, in social networking applications, mapping a user’s followers or friends to their profiles, or in an e-commerce system, mapping products to their categories or attributes.
Efficient Searching and Filtering
HashMaps are useful for checking the existence of elements or performing filtering operations within collections. For instance, checking if a specific key exists or filtering out elements based on certain criteria efficiently.
Optimizing Performance
In cases where performance is crucial and the data set is large, using HashMaps can significantly improve efficiency by allowing constant-time access to elements based on keys.
Features of a HashMap in Java
Java’s HashMap provides the following specific features:
- Key-value pair storage: HashMap stores data in key-value pairs where each key is unique. This structure allows rapid lookup of values based on their associated keys.
- Fast retrieval: Provides constant-time performance (O(1)) for basic operations like
put()
,get()
,containsKey()
andremove()
, assuming a good hash function and minimal collisions. - Resizable: It maintains performance even with an increasing number of elements by automatically resizing itself when the number of elements exceeds a certain threshold (the load factor). This ensures effective memory utilization.
- No order guarantee: The sequence in which entries in a hash map are iterated is not fixed and can vary over time, particularly if the structure is altered by adding or deleting members. If order is important, consider using LinkedHashMap or TreeMap.
- Efficient implementation: HashMap uses an array of Node objects internally to store elements. In case of hash collisions, it uses a linked list (or a balanced tree in Java 8+) to handle multiple entries at the same hash bucket.
- Concurrency: Although Java does not offer thread-safe versions of the HashMap implementation
(java.util.HashMap)
, it does offer synchronized variants such asjava.util.concurrent.ConcurrentHashMap
that allow for concurrent access in multithreaded situations. - Performance tuning: Allows developers to adjust initial capacity and load factor through constructors to optimize for specific use cases or expected data sizes.
- Non-synchronized: Data corruption may result from concurrent access to a HashMap by several threads without synchronization. Use
Collections.synchronizedMap(map)
or ConcurrentHashMap for thread-safe situations.
Java HashMap Constructors
The HashMap class in Java provides a number of constructors for constructing hash map instances with varying starting capacity, load factors, and for copying components from other maps. The HashMap constructors are shown below, along with examples:
HashMap()
The HashMap()
constructor in Java is responsible for creating an empty HashMap, initializing it with a default capacity of 16 and a load factor of 0.75. This means that upon instantiation, the HashMap is initialized without any elements, ready to store key-value pairs.
The initial capacity of 16 signifies the number of buckets in the underlying array structure used to store these pairs. The load factor of 0.75 denotes the threshold at which the HashMap will resize itself. When the number of elements exceeds 75 percent of the capacity, the HashMap automatically increases its size to ensure efficient operation by redistributing the elements and maintaining performance.

HashMap(int initialCapacity)
The HashMap(int initialCapacity)
constructor in Java allows developers to create a HashMap with a user-defined initial capacity and a default load factor of 0.75. This constructor is particularly useful when there is prior knowledge of the approximate number of elements that will be stored in the HashMap. By specifying the initial capacity, developers can allocate the desired number of buckets within the HashMap's internal structure.
Larger initial capacities can reduce the need for resizing, improving performance.

HashMap(int initialCapacity, float loadFactor)
The HashMap(int initialCapacity, float loadFactor)
constructor in Java empowers developers to create a HashMap with both a user-defined initial capacity and a specific load factor. This constructor grants greater control over the HashMap’s resizing behavior based on the anticipated number of elements and the desired threshold for resizing.
It creates a HashMap with the specified initial capacity and load factor. The load factor determines when the HashMap will resize itself to accommodate more elements.

HashMap(Map<? extends K, ? extends V> m)
A Java constructor called “HashMap(Map<? extends K,? extends V> m” makes it easier to create a new HashMap by copying the mappings from a given Map. Using this constructor, you may easily replicate the key-value pairs from an existing Map into a new HashMap.
With this constructor, programmers can quickly duplicate items from a pre-existing Map and combine its contents into a new HashMap. This feature helps when it’s necessary to make a different HashMap with the exact same mappings as an existing Map. It makes it simple to manipulate or change the data without changing the original Map.

Java HashMap Methods
Java HashMap class has a number of methods for carrying out actions including adding items, getting values, determining whether an element already exists, deleting components and more.
These methods provide essential functionalities for adding, retrieving, removing and iterating through elements in a HashMap in Java, enabling efficient manipulation and management of key-value pairs.
Here’s an overview of several key HashMap functions, along with code:
put(K key, V value)
In Java, the put(K key, V value)
function makes it easier to add or remove key-value pairs from the map. This method adds a new key-value association to the HashMap when it is called. The related value is updated with the new value supplied if the supplied key already exists in the HashMap.
If the key was not previously included in the HashMap, this function returns null
. Otherwise, it returns the value that was previously connected with the key before the update.

get(Object key)
Java’s HashMap get(Object key)
function returns the value associated with the given key inside the map. This method returns the value that corresponds to the supplied key when it is invoked. It returns null
if the key can’t be located in the hash map.
This function is essential for retrieving values from the HashMap according to the keys that correspond to those values.

containsKey(Object key)
Java’s HashMap uses the containsKey(Object key)
method to check if a given key is present in the map. This method checks if the HashMap has a mapping for the supplied key when it is called. The function returns true
if the key is present in the HashMap and returns false
otherwise.
This technique is useful for conditional tests to see whether a specific key is present in the HashMap prior to carrying out further actions.

containsValue(Object value)
Java’s HashMap uses the containsValue(Object value)
method to find out if the map contains a given value. When this method is used, it checks to see if the HashMap contains any key-value mappings where the value corresponds to the input value. The method returns false
if the supplied value can’t be found in the HashMap, but returns true
otherwise.

keySet()
Java's HashMap's keySet()
function makes it easier to retrieve a set that contains every key in the map. This method, when called, returns a Set interface, which provides a view of all the keys that are kept in the HashMap.
Developers can iterate, traverse or manipulate the keys independently of the HashMap itself by using the Set
view, which gives them access to the keys stored in the HashMap.

entrySet()
Java’s HashMap entrySet()
function makes it easier to retrieve a Set
that contains all of the key-value pairs that are kept in the map as Map.objects of entry. This function returns a Set
interface with Map when it is invoked.

Java HashMap Example
In this example, we’ll develop a hash map to hold student data along with the relevant age. The example will cover adding elements, getting values back, determining whether a key exists, deleting elements and looping over the HashMap’s contents.
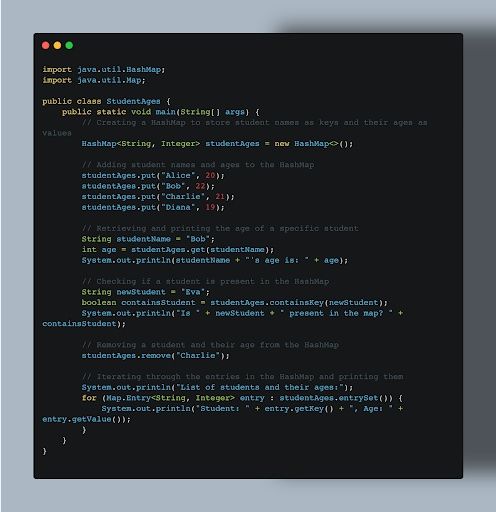
The first part of the code defines a StudentAges
class with a main method, which is the entry point for the program. Inside the main method, a HashMap object called studentAges
is created using the new HashMap<>()
constructor. This essentially creates an empty container for storing student names and their respective ages.
The code then adds entries to the studentAges HashMap using the put method. For example, studentAges.put("Alice", 20)
adds a key-value pair where "Alice"
is the key and 20
is the corresponding value (age). The code also demonstrates checking for a student's presence in the HashMap using the containsKey
method. For example, studentAges.containsKey("Eva")
returns false because "Eva"
is not present in the map. Finally, the code removes a student entry using the remove method and iterates through all remaining entries using a for loop to print them out.
Overall, this code provides a basic example of using a HashMap in Java to store and manipulate student data. It highlights key functionalities like adding, retrieving, checking for presence and removing entries from the map.
How to Create a HashMap in Java
To create a HashMap in Java, you must first instantiate the HashMap class and provide the kinds of keys and values. This is how to make a HashMap:

Let’s create a HashMap to store information about cities and their corresponding populations:
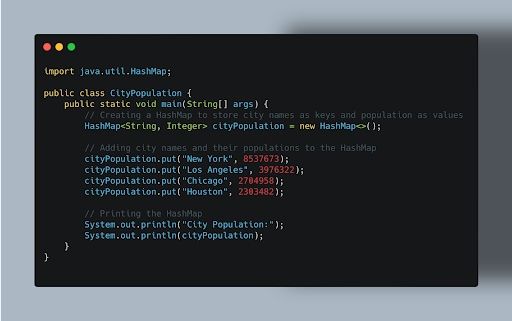
Open the java.util
package and import the HashMap class. To import the HashMap class into another Java file, utilize the import statement to access classes from the java.util
package.
By adding import java.util.HashMap
at the beginning of the Java file where you intend to use the HashMap class, you enable access to the functionalities and capabilities offered by the HashMap data structure.
1. Making a HashMap Instance
To create a HashMap instance, use “HashMap<KeyType, ValueType>”, where KeyType denotes the kind of keys and ValueType denotes the kind of values the map will hold. The instantiation enables the creation of a HashMap instance named mapName
, ready to store key-value pairs conforming to the specified data types. This initialized HashMap offers efficient key-based data organization and retrieval, empowering you to manage and manipulate data efficiently within your Java program.
2. Adding Key-Value Pairs
To add key-value pairs to the HashMap, use the put()
function. For instance, to populate a HashMap called cityPopulation
with city names as keys (strings) and their corresponding population counts (integers), use cityPopulation.put("New York", populationCount)
. This method allows seamless incorporation of key-value pairs into the HashMap, enabling efficient storage and retrieval of city populations based on their associated city names.
3. Printing the HashMap
Use System.out.println(cityPopulation)
to see the contents of the cityPopulation
HashMap. This command prints the actual HashMap object, presenting its key-value pairs in an organized fashion. However, you may use a loop to cycle through the HashMap’s entries or use entrySet()
orkeySet()
in conjunction with a loop to display the keys and their related values individually for a more organized and understandable result.
How to Iterate Through a HashMap in Java
Java’s Map interface offers a variety of ways for iterating across a HashMap. Here are a few methods for iterating over a HashMap:
Using keySet()
keySet()
returns a Set
of keys in the HashMap. Then, you can iterate through these keys and retrieve corresponding values. By using the keySet()
function on a HashMap, you can get a set that contains all of the keys that are kept in the map. Once you have access to each key, you may use a loop to iterate through this Set
. Apply the get()
method to every key in order to obtain and handle the values that correspond to these keys.

Using entrySet()
entrySet()
returns a Set
of key-value pairs (Map.Entry)
in the HashMap. You can iterate through these entries directly. Using a HashMap and the entrySet()
function, you can get a Set
of key-value pairs contained in a Map.objects
of entry. This Set
makes it easier to iterate directly across the key-value pairs in the hash map.
This method uses the entrySet()
function to provide direct access to the keys and their matching values within the HashMap, allowing for quick key-value pair manipulation and processing.

Using forEach()
The forEach()
method allows iteration through the HashMap using a lambda expression, providing key-value pairs for each iteration. This technique makes it easier to iterate through the HashMap by giving you access to key-value pairs at every turn.

HashMap vs. TreeMap vs LinkedHashMap
Here’s a detailed comparison of HashMap, TreeMap and LinkedHashMap.
HashMap
HashMap in Java is a data structure that uses a hash table to store key-value pairs. Basic operations like put()
, get()
and delete()
may be quickly performed using a HashMap, often in constant time. The iteration order is not guaranteed and may change because it does not keep a certain sequence of components.
One null key and many null values are supported by this implementation. It places elements inside using hash codes, and it solves collisions by employing distinct chaining, which entails using linked lists in each bucket. For improved efficiency, a balanced tree is used in place of a linked list in buckets with high collision rates in Java 8 and subsequent versions. When quick key-value search and manipulation are the main goals and ordering is not an issue, HashMaps are frequently utilized.
TreeMap
TreeMap is another Java version that stores key-value pairs in a red-black tree structure. It can be sorted using a custom comparator that was supplied at the time of construction, or it can preserve the keys’ natural ordering.
Because of its balanced tree structure, TreeMap offers log-time performance O(log n)
for fundamental operations. It doesn’t support null keys and allows multiple null values. It arranges components in a bespoke order or according to the natural ordering of their keys.
Moreover, the balanced tree structure of these operations leads to a logarithmic time complexity O(log n)
that maximizes TreeMap’s efficiency while handling large data sets. A TreeMap is useful in situations where sorted traversal or custom key sorting is necessary since iteration across the map adheres to the sorted order of keys. TreeMap is especially useful in situations where sorted traversal or customized key sorting is necessary because of its capacity to preserve components in a set order.
LinkedHashMap
LinkedHashMap combines features of a hash table and a linked list to store key-value pairs in Java. It keeps track of insertion or access order by using an accessOrder
flag that was supplied at the time of creation.
In comparison to HashMap, this version somewhat loses efficiency because it includes a linked list to preserve order. It supports multiple null values and one null key, just like HashMap. Because of the added complexity caused by the linked list, LinkedHashMap’s performance is marginally compromised in comparison to HashMap, even with its hybrid structure.
Like HashMap, LinkedHashMap allows for multiple null values as well as one null key, therefore it maintains compatibility with HashMap’s features while adding ordered storage functionality. LinkedHashMap’s primary feature is its ordered iteration capabilities, which are very helpful when maintaining insertion or access order is important. Depending on the provided accessOrder
flag, iteration over a LinkedHashMap aligns with either the insertion order or the access order.
When maintaining the performance features of the hash table while maintaining insertion or access order preservation, iteration in a LinkedHashMap is advantageous as it follows either the insertion order or the access order. Because of this, LinkedHashMap may be used in situations where ordered iteration is necessary without sacrificing efficiency
Frequently Asked Questions
What is a HashMap in Java?
A HashMap in Java is a data structure that stores key-value pairs, offering fast access to elements based on their keys through hashing mechanisms.
When would you use a HashMap in Java?
HashMap is used when you need efficient key-based retrieval and storage of data without a specific order requirement, suitable for scenarios where quick access to elements is crucial.