Django is a high-level web framework in Python that allows users to easily build web applications rapidly with minimal code. The Django framework follows the model-view-controller (MVC) design pattern. This set-up facilitates easy development of complex database-driven web apps. Through these design patterns, Django emphasizes reusability of components. It also follows the don’t-repeat-yourself (DRY) principle, which reduces repetition in software through abstraction and data normalization to avoid redundant code.
Django can be used in a variety of web applications, including customer relationship management systems (CRMs), social media applications, online marketplaces, on-demand delivery applications and many more. Lots of familiar web applications currently use Django, including Instagram, Dropbox, Spotify, and Mozilla, among several others. As you might suspect based on its widespread use, the MVC design pattern enables rapid development, scalability and security in web applications.
Model-View-Controller
MVC is a popular software design pattern for designing web applications. It uses three interconnected components to separate the internal representations of information from how that information is displayed to a user.
Model
The first element, the model, is the main component of the MVC design pattern. It serves as the web application’s dynamic data structure and is independent of the user interface. The model is also responsible for managing data as well as the logic of the web application. In practice, this structure corresponds to how the web app handles raw data as well as the essential components of the application. For example, if you were to create an app that tracks your runs, the model class would define a “Run” class with corresponding class attributes like miles, pace, time, calories, elevation, heart rate and date.
In the context of Django, a model is a Python class in which each attribute represents a database field. In our example, the miles, pace, time, calories, elevation, heart rate and date attributes are all fields in a database that the model class would define and interact with. The structure of a run class in Django would look like this:
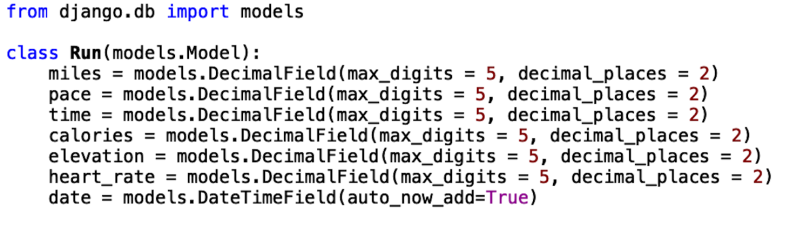
The “Run” class is an abstraction of the details of a run. This is useful because it distills the details of a run into a list that is most relevant to the user. An exhaustive description of a run can include information that may not be relevant to the user. For example, in addition to reporting running distance in miles, distance can be reported in feet, kilometers and yards. This additional information is not essential to the user though. The class allows you to simplify a run in terms of only essential attributes like miles, pace, time, calories, elevation, heart rate and date attributes.
The model element also allows you to normalize data, which helps prevent redundancy and anomalies. Redundancy happens when a database holds the same information in separate locations. For example, if an address field is present in multiple locations, any updates to an address needs to be made in each location. This makes the database error-prone and can result in unreliable/meaningless information. Since the model elements in Django are well defined, though, they prevent data from being duplicated and held in multiple places. Django’s data normalization features also prevent several types of anomalies. For example, it prevents insertion anomalies, where data cannot be inserted due to the absence of other data. It also prevents deletion anomalies, which stops the accidental loss of data due to the deletion of other data.
Further, model elements in Django focus on making web components simple and loosely coupled. Loose coupling in an application means that its parts do not strongly depend on each other. The loose coupling of web components simplifies the life of the developer since they can easily add new features, update existing features and fix bugs without having to rewrite a significant amount of code. This results in faster software updates and a better experience for the user overall.
View
The view code is made up of all of the functions in direct contact with the user. These functions are responsible for making things look nice (UI) and determining the manner in which the user interacts with the app (UX). Views allows you to specify how you would like every page to look. In our run application, you could specify consistent or specific elements such as background colors, text font, navigation bars and footers across each page.
Controller
The controller code connects the models to the views. In practice, this comes down to taking user input at the views level and deciding what to do with it within the models. In the run app example, the controller would take the raw data from the user input at the views level and use the “Run” model class to structure the content of the run. Given this, the controller handles the order of presentation in the view as well as updates the model database with new data.
Scalability
In the current tech landscape, you have to consider scalability when building a web application. Although the number of users for a new app may start small, developers should be appropriately prepared for growth. As your user base grows, so do the number of active visitors and server requests. Django makes scaling easy since it allows you to progressively increase your user base. It has loosely coupled components, which makes debugging bottlenecks easy. For example, using the SQLite3 database, which doesn’t scale well with larger user numbers, instead of MySQL or Postgres database, is a very common source of problems. Fortunately, Django allows you to easily migrate data from SQLite3 databases to more powerful databases like MySQL or Postgres by changing just two to three lines of code. For proof of its utility in scaling, just look at the laundry list of companies that use it, including Spotify, Instagram and YouTube.
Django specifically supports horizontal scaling, which involves spawning up multiple machines to serve smaller parts of the total number of requests. For example, if you have 5,000 requests, instead of serving them on a single machine, you can serve 500 requests each on 10 different machines. Django is useful here because its features allow you to run your application completely stateless, meaning that the server doesn’t store information from one session for future use in another session.
Statelessness allows you to scale apps, decrease the complexity of REST APIs and easily cache results of a request. In Django, you can enable statelessness by modifying it to store data on a remote database, instead of storing data on the default database. The key here is that Django makes running your application on many remote machines easy and straightforward, allowing the application to run statelessly. This is because each new session is independent of client data from past sessions, which eliminates any state dependent issues that may arise. Thanks to these features, apps built with Django can seamlessly scale from 500 to 500,000 users.
Security
Another attractive aspect of Django is that it comes with many free, built-in security features. One protection that Django provides is against cross-site scripting (XSS), thereby protecting the user’s browser. Django also protects against cross-site request forgery (CSRF), which prevents attackers from performing actions using the credentials of another user without that user’s knowledge or consent. Finally, Django protects against SQL injection, which stops unauthorized data deletion or data leakage.
Django also has significantly more built-in protections compared to other popular frameworks. For example, Flask is another popular web framework that relies on third-party software for the same protections. This puts more pressure on the software development team to monitor security by making sure the third party software is up to date. Since Django comes with most protections built-in for free, it is much easier to secure your applications upfront and monitor security during the lifetime of the application. If you are interested in reading further, you can read the Django documentation on security, Security in Django.
Django in Use: Instagram
Now that we’ve seen some of the benefits of Django, let’s look at a specific instance of its application in practice. The quality of Instagram’s user experience and service is in large part due to much of its core functionality being written in Django. For example, Instagram uses the Django application Sentry for error reporting. This allows Instagram developers to diagnose bugs, modify code and optimize the performance of application components. Instagram is also built on top of a Django tool called Dynostats, which also allows you to monitor efficiency of application components. Using Dynostats, developers can monitor user requests, application responses, application latency and more.This allows developers to easily pin down the causes of an application slowing down and which lead to solutions faster.
Put Django to Work for You
Through following the MVP design principle, the Django framework enables rapid development, scalability and security in web applications. The modular architecture of models in Django makes it easy to update/maintain specific components of your web applications, while leaving other components safely unchanged. Django allows companies to not only quickly develop web components for their applications, but also to quickly make small changes to increasing complex code down the road. Further, Django prevents most common security breaches, which leads to additional savings in development time. All in all, Django is a fantastic web framework that takes out much of the hassle of building a web application by enabling fast development through the MVP design, ensuring security and enabling scalability.