Technical coding questions are some of the most intimidating aspects of the software development interview process. Though the questions may look quite different for different companies, most companies subject interviewees to some version of it.
Coding interview questions aren’t meant to be tools of terror. Each of the questions interviewers ask is trying to gauge something specific about candidates, whether it’s simple coding prowess, systems knowledge, familiarity with design principles, or just the ability to collaborate with others — including the interviewer.
Built In asked three individuals familiar with the software engineering recruitment process to talk about nine common coding interview questions and the skill sets they are measuring for. Questions were sourced from popular practice coding interview websites such as LeetCode, as well as coding interview prep textbooks.
Interviewers sometimes have different opinions about coding problems and their effectiveness in testing for specific skills, but one thing is clear — companies want candidates who engage with interviewers, ask for clarification, and don’t give up. Just work with the interviewer and let all your knowledge and creativity shine through. Most likely, that’s exactly what they’re looking for in a candidate.
9 Common Coding Interview Questions
- Merge two sorted linked lists and return it as a sorted list.
- Given a roman numeral, convert it to an integer.
- Given an array of integers, every element appears twice except for one. Find that one.
- Given a binary tree, print the bottom view from left to right.
- Given an expression string x, examine whether the pairs and the orders of “{”,“}”,“(”,“)”,“[”,“]” are correct in the expression. For example, the function should return “true” for “[()]{}{[()()]()}” and “false” for “[(])”.
- Given two numbers M and N, find the position of the rightmost different bit in the binary representation of numbers.
- What’s the runtime (BigO) of the following code?
- Design a parking lot using object-oriented principles.
- If you were designing a web crawler, how would you avoid getting into infinite loops?
Merge two sorted linked lists and return it as a sorted list.
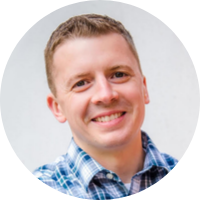
Josh Tucholski, director of curriculum and instruction at coding bootcamp Tech Elevator
What skillset is this testing for? A lot of the time, you’re not necessarily encountering a specific problem with a linked list. But you are encountering problems like what this is asking, where perhaps the developer has to get data from two separate APIs and find some way to present it together in a sorted fashion. They might be solving the exact same problem, just not using a linked list. We can also generalize this problem by saying, “Alright, we have two lists of numbers that are sorted. How would we go about producing a third one where it includes all of them?”
Is this a good interview question? There’s yet to be a scenario in my professional development career where I’ve encountered a linked list, but the algorithm is what’s really important here. If I gave you two things in sorted order, could you find a way to merge them in another sorted order?
Some companies that are maybe not as familiar with alternative education options for software development, or that are more fundamentally rooted in solving deep algorithmic problems, might leverage questions like this. In the markets that we’re in at Tech Elevator in the Midwest, we actually don’t have a lot of questions like this. Out of a class of 40 or 50 students, there might be one student that has been given a question with a linked list, for example.
Given a roman numeral, convert it to an integer.
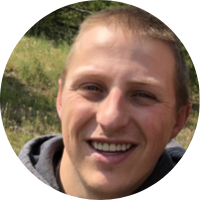
Cody Daig, technical mentor at Galvanize
What skillset is this testing for? Once you see the solution, it can be very easy to figure out — however, getting to that solution is often a struggle for most people. Because you have to think not only about where you’re at, but the next value in that Roman numeral. So, for example, four is “IV.” And so you can’t just go, “‘I,’ that’s one, ‘V,’ that’s five — boom, we’re at six!” Actually, you’re at four. So how do you differentiate between adding and subtracting not only looking at where you’re at but where you’re going? So it’s kind of got those two different pieces there.
Is this a good interview question? It depends on the knowledge of the interviewee going into the question here. I make the assumption that most of the time people know Roman numerals, and that can be an incorrect assumption, because not everyone actually knows or understands Roman numerals. So if you are coming into it not even knowing Roman numerals, it’s a challenging one. In general, I would say you’re not at that much more of a disadvantage, as long as you’re upfront and honest, like, “Hey, I’ve never worked with Roman numerals before.”
Given an array of integers, every element appears twice except for one. Find that one.
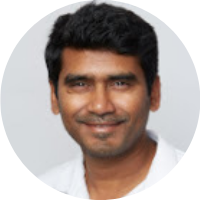
Anil Kadimisetty, director of engineering at Chainalysis
What skillset is this testing for? Not every interview candidate who gets selected actually solves a problem. That actually doesn’t matter that much. Because in the real world, a single individual does not know the answers to everything they’re trying to solve. You are going to only succeed when you’re able to collaborate with others, get others feedback and act on that. When you get stuck, what do you do? If someone is giving you a hint, are you responding well to the hint? That means you’re probably great at collaborating later on when you come here.
Is this a good interview question? At Chainalysis, we’re about cryptocurrency. In an interview, if we ask about that — because that’s what we do on a day-by-day basis — most people would fail, because no one really knows about cryptocurrencies.
So what you do is, you use the most abstract way of asking about those things without mentioning the topics, which are domain specific. If you abstract the domain knowledge out of it, you will come down to a basic problem like this. So that’s what this is. It’s actually making the hiring bar common across all candidates with different domain experiences. This is a good equalizer.
Given a binary tree, print the bottom view from left to right.
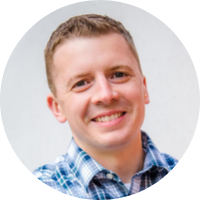
Josh Tucholski, Tech Elevator
What skillset is this testing for? A lot of people will bring up trees when they talk about database structure and design — it’s not like tables and keys, it’s more like underlying indexes and how those get managed. You’re getting into the realm of a database administrator, or a database developer who’s very specialized into fine-tuning databases.
If I had a student encounter this problem, I’d encourage them to work with the interviewer, rather than just giving up. They might want to basically go down into the level of how they would structure this problem in their code. They might talk about classes that they might use or various other data collections that they’ve worked with and explain how they might solve the problem.
Is this a good interview question? Someone who’s entering into a junior position often lacks prior experience professionally, where they can stand out and say, “Here is what I’m capable of doing.” And so we sometimes default to these questions to demonstrate that candidates have a foundational understanding? Unfortunately, these questions assume that one has had a foundational computer science education.
There are other ways to measure that. Have them either speak through a project that they specifically worked on, or give them some code and ask them to explain it to you, because that gets at their communication capability to explain what code is doing. When they walk through code, do they gloss over a number of things? Or do they get very specific?
Given an expression string x, examine whether the pairs and the orders of “{”,“}”,“(”,“)”,“[”,“]” are correct in the expression. For example, the function should return “true” for “[()]{}{[()()]()}” and “false” for “[(])”.
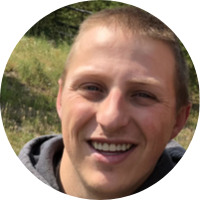
Cody Daig, Galvanize
What skillset is this testing for? All that you use to solve this problem is the stack. Once you realize you should be using a stack, I would say it’s a pretty straightforward question. Getting to think in that style of using a different data structure than you might think to solve it, that’s the challenge.
What you do is, when you see an open parentheses, square bracket or curly brace, you add that to the stack. And then when you see a closing side of the pair, then you need to pop from the stack. And if the closing matches the opening, good. If it doesn’t, then it’s incorrect or invalid.
Is this a good interview question? It’s actually one of my favorite problems. Thinking about it from the data structures perspective, I think that it’s a very practical skill — although you’re not necessarily going to be writing algorithms to see if parentheses are balanced. But applying knowledge to solve the problem, that’s the key here. Realizing that there are data structures out there to help solve this problem really easily, that are often used when actually building applications — and making sure that you’re using the most efficient data structure possible to accomplish whatever you’re trying to accomplish.
Given two numbers M and N, find the position of the rightmost different bit in the binary representation of numbers.
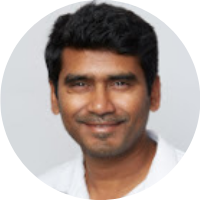
Anil Kadimisetty, Chainalysis
What skillset is this testing for? It’s useful when you are doing work on low-level systems. Because when you’re working at a very low level, you need to understand how things are represented in actual physical memory. Like when you type your name, how is this saved on the disk? How is this saved in memory? That’s all saved in the binary representation of the numbers. If you understand that, you can write the part of code that is actually doing the work to save information in memory in a very proper and efficient way.
Is this a good interview question? There are a lot of things that need low-level stuff. Let’s say you are making an iPhone — not an iPhone app — you’re going very low level at that point. If you understand how to solve this problem, if you can have a discussion around the topic, we can assume that you’re good at low-level stuff. That’s kind of where it’s useful. So this is not super applicable to every company, but it’s useful to companies that do a lot more low-level stuff.
What’s the runtime (BigO) of the following code?
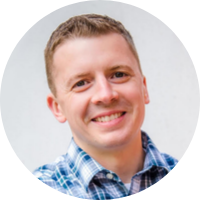
Josh Tucholski, Tech Elevator
What skillset is this testing for? Another common term that’s used is “algorithmic complexity.” The question could be: “If I’m going to give someone a block of code, can you tell me what are the worst-case scenarios that I have to consider?” Because that’s what BigO and algorithmic complexity really mean.
That’s kind of a cue to the interviewee to think about, “What are some of the worst-case or edge-case scenarios that I have to be aware of?” And that’s important, because those things, while they account for maybe one or two percent of the number of inputs, they’re the kinds of things that often will crash our programs.
Is this a good interview question? As software developers, we all have a tendency, when given a problem, to immediately jump into all of the “what ifs” and the edge cases that we have to worry about — especially after we’ve been kind of burned a couple times. We do tend to try and problem solve and prevent those worst-case scenarios.
Being able to ask in plain English, “What is this code doing and what’s the worst-case scenario?” is an easier way to communicate than to recall the difference between BigO(n) or linear or logarithmic or n2 or exponential time, because then you’re counting on someone having been exposed, again, to that type of formal education that covered that content.
Design a parking lot using object-oriented principles.
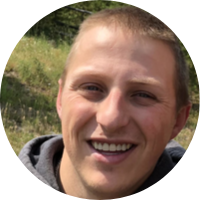
Cody Daig, Galvanize
What skillset is this testing for? Here we’re looking at, “Can you think through how to take something that’s not obviously an object at first, and turn it into a series of objects to accomplish what you’re trying to accomplish?” This will vary a little by language, but ultimately it’s a class. It’s a problem to get you to think through and break down classes.
Sure, you have the parking lot and you have parking spaces. But if we have a programming need for this, we probably need to think through, is a space occupied? That means you have to have the concept of a class of a vehicle, and the vehicle has to occupy a space. You can have the concept of entrances, and parking lots can even have more than one floor. So it really comes down to whether you can take this problem and break it down and think through all of the possible things that need to be considered when building a parking lot.
Is this a good interview question? It’s very common to have a question that is vague, but it’s also acceptable for the interviewee to come back and ask questions. “What are you looking for out of this problem? Are we talking a parking lot at Walmart? Are we talking a parking lot with multiple floors?” You can ask questions to determine how complex you need to make this. But it’s a very valid piece for them to just see your thought process and things that you might need to take into consideration.
If you were designing a web crawler, how would you avoid getting into infinite loops?
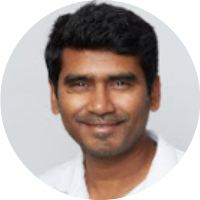
Anil Kadimisetty, Chainalysis
What skillset is this testing for? There are two angles for this question. One angle is, can you design a system to solve a business problem? You’re breaking down a problem into small pieces, like how to follow steps to solve problems, how to build a system with various components in it, which is how we solve real-life applications.
Is this a good interview question? For this one, you’re not generally expecting to ask a question that the candidate is hearing for the first time. We want something they already know — they should probably know the answer. We want to have a discussion about, what are the various ways to solve this problem? What are the best practices? Because it will tell us how much they’ve been learning, and it shows how they learn new things and keep up to date with what’s going on.
Responses have been edited for length and clarity.