In this article, we will explore the $(document).ready method equivalent in JavaScript without using jQuery. The $(document).ready() method in jQuery is used to execute JavaScript code as soon as the document object model (DOM) ready. It ensures that the DOM is fully loaded before executing any code that manipulates the DOM.
2 Document Ready Methods in JavaScript
DOMContentLoaded
Method: In this method, theDOMContentLoaded
event initiates only once the HTML has been parsed and loaded.Readystatechange
Method: This schedules an event to trigger only once the ready state of the document is set to “complete.”
This method only waits for the DOM to be loaded, without taking into account the loading of style sheets, images and iframes. This method specifies the function to execute when the DOM is fully loaded. There are a few ways by which similar functionality can be achieved without jQuery, including:
- By implementing the
DOMContentLoaded
event. - By using the async/await function with the
'load'
event.
How to Use Document Ready in JavaScript
DOMContentLoaded Event Method
We will use the DOMContentLoaded
method to update the content of an HTML element as soon as it is ready. The DOMContentLoaded
event fires when the initial HTML document has been completely parsed and loaded. It does not wait for stylesheets and images to finish loading.
This event gets executed once the basic HTML document is loaded and its parsing has taken place. Some of the advantages of using the DOMContentLoaded
event, include:
- It helps in improving user experience as it shows messages or content much faster.
- It takes less time to load the page.
DOMContentLoaded Event Syntax
document.addEventListener("DOMContentLoaded", function(e) {
console.log("Example page has loaded!");
});
DOMContentLoaded Event Example
In this example, we are displaying a heading using the tags. The content of the heading will be displayed only when the HTML document has been completely loaded. This is ensured with the help of the DOMContentLoaded
method.
<!DOCTYPE html>
<html>
<head>
<title>DOM Content Loaded Example</title>
<script>
document.addEventListener('DOMContentLoaded', () => {
const greeting = document.querySelector('#greeting');
greeting.textContent = 'Example';
});
</script>
</head>
<body>
<h1 style="color: blue;" id="greeting"></h1>
<h3>$(document).ready equivalent without jQuery</h3>
</body>
</html>
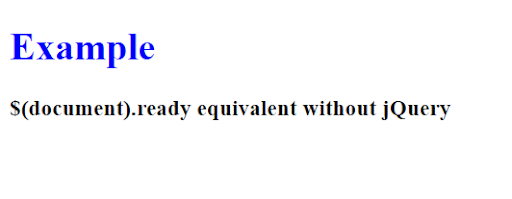
The JavaScript code inside the tags is executed when the initial DOMContentLoaded
event is triggered. This event is fired when the initial HTML document has been completely loaded and parsed, without waiting for external resources like images and stylesheets.
As a result, when the DOMContentLoaded event occurs, the element with the ID greeting
will display the text “Example”
instead of being empty.
This demonstrates how to manipulate the content of an HTML element using Javascript when the DOMContentLoaded
event is triggered.
Readystatechange Method
Thereadystatechange
event is triggered when the ready state of the document changes. By checking if the document.readyState
is set to “complete,”
you can determine when the DOM is ready.
Readystatechange Method Syntax
document.onreadystatechange = function() {
if (document.readyState === "complete") {
console.log("Page is loaded completely!");
}
};
Readystatechange Example
In this example, we are using the readystatechange
event and checking the document.readyState
property to determine when the DOM is ready.
The readystatechange
event is triggered when the ready state of the document changes. The document.readyState
property represents the current state of the document and can have different values throughout the loading process.
The document.readyState
property can have the following values:
“loading”
: This means the document is still loading.“interactive”
: The DOM is loaded, but some external resources are still loading.“complete”
: The DOM is completely loaded.
By using the document.onreadystatechange
event and checking the document.readyState
property, we can accurately detect when the DOM is ready and perform necessary actions or execute code accordingly. In this example, we are changing the background color of the “Click Me” button to red and the text to white on page load.
<!DOCTYPE html>
<html>
<head>
<title>onreadystatechange Example</title>
</head>
<body>
<h1 style="color: blue;">Example</h1>
<h3>$(document).ready equivalent without jQuery</h3>
<button id="myButton">Click me!</button>
</body>
<script>
document.onreadystatechange = function () {
if (document.readyState === "loading") {
console.log('Page is loading');
}
if (document.readyState === "interactive") {
console.log('DOM is ready');
}
if (document.readyState === "complete") {
console.log('Page is fully loaded');
const button = document.getElementById('myButton');
button.style.backgroundColor = 'red';
button.style.color = 'white';
}
};
</script>
</html>
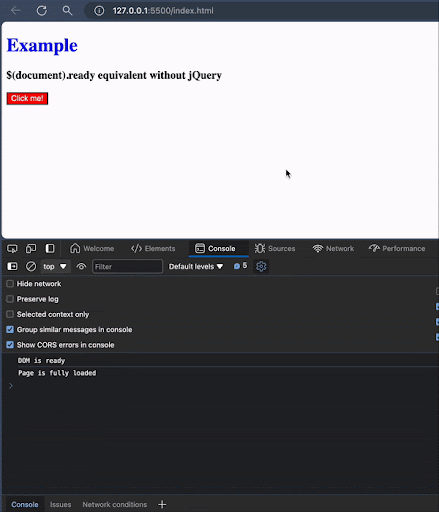
Document Ready Method Supported Browsers
- Google Chrome 90.0+
- Internet Explorer 9.0
- Safari 4.0
- Opera 10.5
- Firefox 3.6
And that’s how you can use the $(document).ready
method equivalent without using jQuery.
Frequently Asked Questions
How do you use document ready in JavaScript?
There are two common ways to use the document ready method in JavaScript:
DOMContent Loaded
Event Method.Readystatechange
method.
How do you write the document ready function in JavaScript?
- The document ready function using the DOMContentLoaded Event method follows this syntax:
document.addEventListener("DOMContentLoaded", function(e) { console.log("Example page has loaded!"); });
- The readystatechange method follows the syntax:
document.onreadystatechange = function() { if (document.readyState === "complete") { console.log("Page is loaded completely!"); } };