There are several situations in which you’ll need to convert a JavaScript string into a number. It’s useful for comparing numerical data that comes in a string format, completing math operations on a string and more.
7 Methods to Convert a JavaScript String to a Number
- Using
parseInt()
- Using
number()
- Using the unary operator
(+)
- Using
parseFloat()
- Using
Math.floor()
- Multiply with number
- Using the double tilde
(~~)
operator
Below are several different methods you can use to convert a string into a number in JavaScript with example code.
7 Ways to Convert a JavaScript String to a Number
1. Using parseInt()
parseInt()
parses a string and returns a whole number. Spaces are allowed. Only the first number is returned.
This method has a limitation though. If you parse the decimal number, it will be rounded off to the nearest integer value and that value is converted to string. One might need to use the parseFloat()
method for literal conversion.
myString = '129'
console.log(parseInt(myString)) // expected result: 129
a = 12.22
console.log(parseInt(a)) // expected result: 12
2. Using Number()
Number()
can be used to convert JavaScript variables to numbers. We can also use it to convert the string into a number. If the value can’t be converted to a number, NaN
is returned.
Number("10"); // returns 10
Number(" 10 "); // returns 10
Number("10.33"); // returns 10.33
3. Using Unary Plus Operator (+)
The unary plus operator (+)
precedes its operand and evaluates to its operand, but it also attempts to convert it into a number, if it isn't already.
const x = 25;
const y = -25;
console.log(+x); // expected output: 25
console.log(+y); // expected output: -25
console.log(+''); // expected output: 0
4. Using parseFloat()
parseFloat()
parses a string and returns a number. Spaces are allowed. Only the first number is returned.
parseFloat("10"); // returns 10
parseFloat("10.33"); // returns 10.33
parseFloat("10 20 30"); // returns 10
parseFloat("10 years"); // returns 10
parseFloat("years 10"); // returns NaN
5. Using Math.floor()
The Math.floor()
function returns the largest integer less than or equal to a given number. This can be a little tricky with decimal numbers since it will return the value of the nearest integer as a whole number.
str = '1222'
console.log(Math.floor(str)) // returns 1222
a = 12.22
Math.floor(a) // expected result: 12
6. Multiply with number
Multiplying the string value with the 1
won’t change the value, and it will convert the string to a number by default.
str = '2344'
console.log(str * 1) // expected result: 2344
7. Double tilde (~~) Operator
We can use the double tilde (~~)
operator to convert the string to number.
str = '1234'
console.log(~~str) // expected result: 1234
negStr = '-234'
console.log(~~negStr) // expected result: -234
How to Pick the Best Method to Convert a JavaScript String to a Number
Although there are many ways to convert string to number in JavaScript, one has to be careful which one they use.
1. Check for Null Explicitly
If null represents a special case in your application, it’s important to check for it before converting.
Number(null) // result: 0
parseInt(null) // result: NaN
2. Fallback Values
Use logical OR (||)
to provide a fallback value when conversions return NaN
. For example:
`const num = Number(str) || 0;. `
3. Readability
I prefer to use the Number()
method because it’s easier for developers to read the code, and it’s quite intuitive to realize that it’s converting a string to Number
.
4. Performance
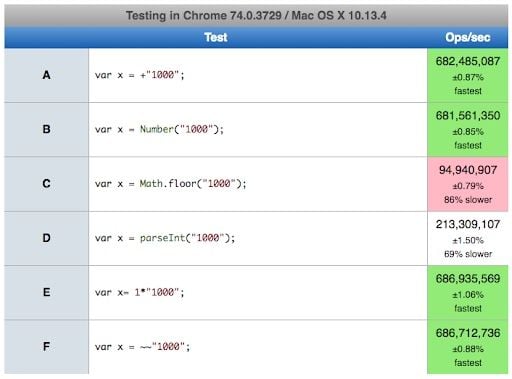
Above is the performance chart for each method. Sometimes it makes sense to select the method with the highest performance.