The URLSearchParams
API provides a way to retrieve and work with the data in the URL query parameters. Here’s everything you need to know about how it works and how to use it in JavaScript.
12 URLSearchParams Methods
Get
GetAll
Has
toString
Append
Set
Entries
Keys
Values
forEach
Sort
Delete
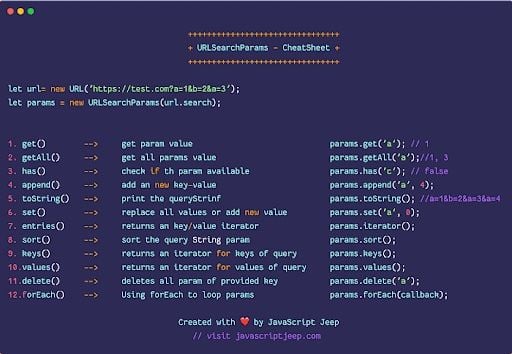
3 Ways to Create URLSearchParams Objects
We can create a URLSearchParams
object in three different ways.
1. Using URL Object
The first way is to use the URL
object.
let url= new URL('https://javascriptjeep.com?mode=night&page=2');
let params = new URLSearchParams(url.search);
2. Using Query String
The second method is using a query string:
params = new URLSearchParams("mode=night&page=2");
// you can also have only query string
var params = new URLSearchParams("?mode=night&page=2");
3. Using Entry
The third method is using entry:
params= new URLSearchParams([["mode", "night"],["page", "2"]]);
//pass object
params = new URLSearchParams({mode: "night", page : "2"});
12 URLSearchParams Methods
In this section we’ll cover 12 ways to use URLSearchParams. For each example, we’ll be using the URL
:
let url= new URL('https://test.com?mode=night&page=2&mode=day');
let params = new URLSearchParams(url.search);
1. Get
Get
allows you to get the first value associated from the given search parameter. If the param isn’t found then null
will be returned.
let mode = params.get('mode'); // night
let time = params.get('time'); // null
2. GetAll
getAll
retrieves all the values of the search parameter as an array.
let modes = params.getAll('mode'); // ["night", "day"]
3. Has
has
checks if a parameter with the specified name exists:
let hasMode = params.has('mode'); //true
let hasTime = params.has('time'); //false
4. toString
toString
will print the query string:
params.toString(); //mode=night&page=2&mode=day
5. Append
The append
method appends a new key-value to the search params.
params.append('tag', "js");
params.toString(); //mode=night&page=2&mode=day&tag=js
If the same key is appended multiple times, it will appear in the parameter string multiple times for each value.
params.append('tag', "tricks");
params.toString(); //mode=night&page=2&mode=day&tag=js&tag=tricks
6. set
Sets the value of the search parameter to the given value.
params.set('page', 3);
params.toString(); // mode=night&page=3&mode=day&tag=js&tag=tricks
If there were several matching values, this method deletes the others.
params.set('tag', "javascript")
params.toString(); // mode=night&page=3&mode=day&tag=javascript
If the search parameter doesn’t exist, this method creates it.
params.set('n',"J") //mode=night&page=3&mode=day&tag=javascript&n=J
7. entries
entries
returns an iterator
allowing iteration through all key/value pairs.
console.log(...params.entries());
// you can also use in loop
for(var [key, value] of params.entries()) {
console.log(key+ ' => '+ value);
}
mode => night
page => 3
mode => day
tag => javascript
n => j
8. keys
keys
returns an iterator for keys of the search params.
console.log(...params.keys()); // mode page mode tag n
9. values
values
returns an iterator for values of the search params.
console.log(...params.values()); // night 3 day javascript j
10. forEach
Using forEach
, you can loop through the params.
params.forEach(function(value, key) {
console.log(key, value);
});
11. sort
sort
sorts the key/value pairs.
params.sort();
params.toString();// mode=night&mode=day&n=j&page=3&tag=javascript
12 . Delete
This deletes the given search parameter and all its associated values from the list of all search parameters.
params.delete('mode');
params.toString(); // name=Jeep&page=3&tag=javascript